DataObjectBase Class Reference
[Model]
Base class for all Data Access Objects. More...
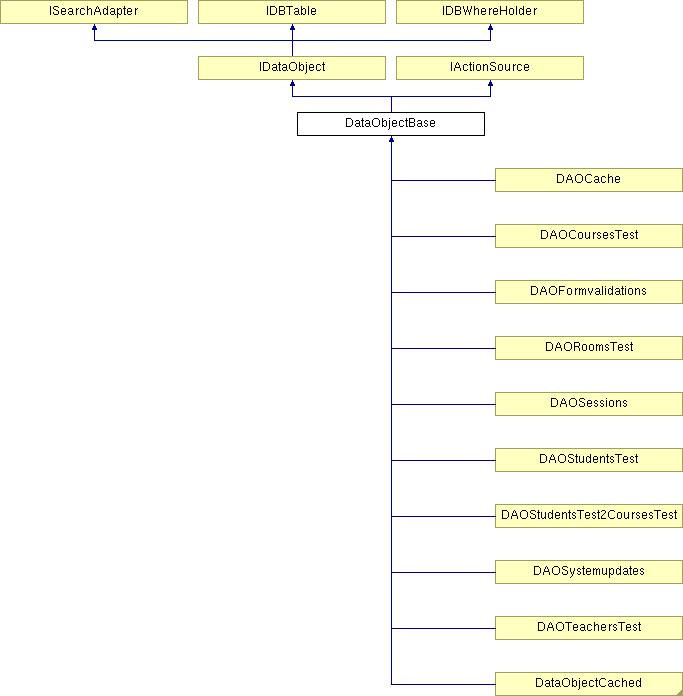
Public Member Functions |
|
__clone () | |
__construct () | |
__destruct () | |
__sleep () | |
__toString () | |
__wakeup () | |
add_where ($column, $operator=null, $value=null, $mode= 'AND') | |
Adds where to this. |
|
add_where_object (IDBWhere $where) | |
Adds IDBWhere instance
to this. |
|
apply_modifier ($modifier) | |
Apply a modifier. |
|
count () | |
Count resulting items. |
|
create_count_query ($policy=self::NORMAL) | |
Prepare a count query. |
|
create_delete_query ($policy=self::NORMAL) | |
Prepare a delete query. |
|
create_insert_query () | |
Prepare an insert query. |
|
create_replace_query () | |
Prepare an insert query. |
|
create_select_query ($policy=self::NORMAL) | |
Prepare a select query. |
|
create_update_query ($policy=self::NORMAL) | |
Prepare an update query. |
|
delete ($policy=self::NORMAL) | |
Delete this object. |
|
equals ($other) | |
Returns true if a both this and the given
object are identical. |
|
execute () | |
Implemented from ISearchAdapter.
|
|
fetch () | |
fetches next row into this objects var's
|
|
fetch_array () | |
Fetch and return an array. |
|
find ($policy=self::NORMAL) | |
Find results, either normal or crosstable.
|
|
find_array () | |
Return array of elements, based on current
configuration. |
|
get ($column_or_value, $value=null) | |
Finds and fetches first result of a select
for $value on $column_or_value. |
|
get_action_source_name () | |
Identify for generic actionh processing.
|
|
get_actions ($user, $context= 'view', $params=false) | |
Get all actions. |
|
get_filters () | |
Return array of filters. |
|
get_matching_relations (IDBTable $other) | |
Returns relations between two tables.
|
|
get_sort_default_column () | |
Get the column to sort by default. |
|
get_sortable_columns () | |
Return array of sortable columns. |
|
get_table_alias () | |
Returns alias of table, if any. |
|
get_table_alias_escaped () | |
Returns alias of table, if any - but
escaped. |
|
get_table_constraints () | |
Returns array of constraints. |
|
get_table_driver () | |
Returns DB driver fro this
table. |
|
get_table_field ($column) | |
Returns field fpr given column. |
|
get_table_fields () | |
Returns array of columns. |
|
get_table_keys () | |
Returns array of keys. |
|
get_table_name () | |
Returns name of table. |
|
get_table_name_escaped () | |
Returns name of table, but escaped. |
|
get_table_relations () | |
Returns array of relations. |
|
get_wheres () | |
Returns root collection of wheres. |
|
insert () | |
Insert data. |
|
is_same_as ($other) | |
Returns true if a both this and the given
object refer to the same instance in the DB.
|
|
join (IDBTable $other, $conditions=array(), $type=DBQueryJoined::INNER) | |
Join another table to this. |
|
limit ($start=0, $number_of_items=0) | |
Limit result. |
|
query ($query, $policy=self::NORMAL, $connection=false) | |
Execute a query (SELECT). |
|
quote ($val) | |
Quote and escape a string. |
|
read_from_array ($values) | |
read properties of object form array
|
|
register_query_hook (IDataObjectQueryHook $hook) | |
Register hook to be called for every query
created. |
|
replace () | |
Inserts if data does not exist, updates
else. |
|
save () | |
Either update or insert object. |
|
set_default_values () | |
Sets all default values on instance.
|
|
set_table_alias ($alias) | |
Set a table alias for this instance.
|
|
sort ($column, $order=self::ASC) | |
Sort result by colum. |
|
to_string () | |
unset_internals ($arr_properties) | |
Removes all properties from array that are
marked using DBField::INTERNAL. |
|
update ($policy=self::NORMAL) | |
Update current item. |
|
validate () | |
Validate this object. |
|
Protected Member Functions |
|
configure_count_query ($query, $policy) | |
Configure a count query. |
|
configure_delete_query ($query, $policy) | |
Configure a delete query. |
|
configure_insert_query ($query) | |
Configure an insert query. |
|
configure_replace_query ($query) | |
Configure an insert query. |
|
configure_select_query ($query, $policy) | |
Configure a select query. |
|
configure_update_query ($query, $policy) | |
Configure an update query. |
|
create_table_object () | |
Create the table object describing this
dataobejcts table. |
|
execute_query_hooks ($query) | |
Run hooks. |
|
find_action_command ($name) | |
Resolve action name to command. |
|
get_actions_for_context ($context, $user, $params) | |
To be overloaded. |
|
get_field_values ($only_set=true) | |
Returns aoociative array of fields and
values. |
|
get_properties_to_exclude_for_sleep () | |
init_table_object () | |
Init table (create, retrieve) DBTable
instance. |
|
replace_manual () | |
Inserts if data does not exist, updates
else. |
|
reset () | |
set_last_insert_id ($connection) | |
Set the last insert id if there is an
autoincrement INTEGER field. |
|
Protected Attributes |
|
$joins = array() | |
$limit = array(0,0) | |
$order_by = array() | |
$queryhooks = array() | |
$resultset = null | |
$table = null | |
$where = null |
Detailed Description
Base class for all Data Access Objects.
Behaves somewhat like DB_Dataobject
Definition at line 10 of file dataobjectbase.cls.php.
Constructor & Destructor Documentation
DataObjectBase::__construct | ( | ) |
Definition at line 45 of file dataobjectbase.cls.php.
00045 { 00046 $this->table = $this->init_table_object(); 00047 $this->where = new DBWhereGroup($this); 00048 }
DataObjectBase::__destruct | ( | ) |
Definition at line 69 of file dataobjectbase.cls.php.
Member Function Documentation
DataObjectBase::__clone | ( | ) |
Definition at line 78 of file dataobjectbase.cls.php.
00078 { 00079 $this->resultset = null; 00080 $this->where = new DBWhereGroup($this); 00081 $this->limit = array(0,0); 00082 $this->order_by = array(); 00083 }
DataObjectBase::__sleep | ( | ) |
Definition at line 85 of file dataobjectbase.cls.php.
00085 { 00086 $properties = get_object_vars($this); 00087 foreach($this->get_properties_to_exclude_for_sleep() as $prop) { 00088 unset($properties[$prop]); 00089 } 00090 return array_keys($properties); 00091 }
DataObjectBase::__toString | ( | ) |
Definition at line 102 of file dataobjectbase.cls.php.
00102 { 00103 return $this->to_string(); 00104 }
DataObjectBase::__wakeup | ( | ) |
Definition at line 97 of file dataobjectbase.cls.php.
00097 { 00098 $this->table = $this->create_table_object(); 00099 $this->where = new DBWhereGroup($this); 00100 }
DataObjectBase::add_where | ( | $ | column, | |
$ | operator = null , |
|||
$ | value = null , |
|||
$ | mode = 'AND' |
|||
) |
Adds where to this.
Example:
$object = new mytable(); $object->add_where('id', '>', 3); $object->add_where('name', 'in', array('Hans', 'Joe')); $object->add_where("(email like '%provider1%' or email like '%provider2%')");
Will query using the following SQL:
SELECT * FROM mytable WHERE (id > 3 AND name IN ('Hans', 'Joe') AND (email like 'provider1' or email like 'provider2'));
- Parameters:
-
string $column Column to query upon, or a full sql where statement string $operator Operator to execute mixed $value Value(s) to use string Either DBWhere::AND or DBWhere::OR
Implements IDBWhereHolder.
Definition at line 497 of file dataobjectbase.cls.php.
DataObjectBase::add_where_object | ( | IDBWhere $ | where | ) |
Adds IDBWhere instance to this.
Implements IDBWhereHolder.
Definition at line 504 of file dataobjectbase.cls.php.
00504 { 00505 $this->where->add_where_object($where); 00506 }
DataObjectBase::apply_modifier | ( | $ | modifier | ) |
Apply a modifier.
- Parameters:
-
IDBQueryModifier The modifier to be applied
Implements ISearchAdapter.
Definition at line 660 of file dataobjectbase.cls.php.
DataObjectBase::configure_count_query | ( | $ | query, | |
$ | policy | |||
) | [protected] |
Configure a count query.
- Parameters:
-
DBQuerySelect $query int $policy
Definition at line 1115 of file dataobjectbase.cls.php.
01115 { 01116 $this->configure_select_query($query, $policy); 01117 }
DataObjectBase::configure_delete_query | ( | $ | query, | |
$ | policy | |||
) | [protected] |
Configure a delete query.
- Parameters:
-
DBQueryDelete $query int $policy
Definition at line 1073 of file dataobjectbase.cls.php.
01073 { 01074 $where = new DBWhereGroup($query->get_table()); 01075 $where->add_where_object($this->where); 01076 01077 if (!Common::flag_is_set($policy, self::WHERE_ONLY)) { 01078 $fields = $this->get_field_values(); 01079 foreach($this->get_table_keys() as $column => $field) { 01080 if (!isset($fields[$column])) { 01081 // Prevent unwanted mass updates... 01082 throw new Exception(tr('Trying to DELETE without all keys set. If intended, use WHERE_ONLY policy', 'core')); 01083 } 01084 $where->add_where($column, '=', $this->$column); 01085 } 01086 } 01087 01088 $query->add_where_object($where); 01089 01090 $query->set_limit($this->limit[0], $this->limit[1]); 01091 foreach($this->order_by as $order_by) { 01092 $query->add_order(key($order_by), current($order_by)); 01093 } 01094 }
DataObjectBase::configure_insert_query | ( | $ | query | ) | [protected] |
Configure an insert query.
- Parameters:
-
DBQueryInsert $query
Reimplemented in DAOCache.
Definition at line 983 of file dataobjectbase.cls.php.
00983 { 00984 $fields = $this->get_field_values(); 00985 //foreach($this->get_table_keys() as $column => $field) { 00986 // unset($fields[$column]); 00987 //} 00988 00989 $query->set_fields($fields); 00990 }
DataObjectBase::configure_replace_query | ( | $ | query | ) | [protected] |
Configure an insert query.
- Parameters:
-
DBQueryInsert $query
Definition at line 1049 of file dataobjectbase.cls.php.
01049 { 01050 $fields = $this->get_field_values(); 01051 $query->set_fields($fields); 01052 }
DataObjectBase::configure_select_query | ( | $ | query, | |
$ | policy | |||
) | [protected] |
Configure a select query.
- Parameters:
-
DBQuerySelect $query int $policy
Definition at line 924 of file dataobjectbase.cls.php.
00924 { 00925 $query->add_where_object($this->where); 00926 00927 if (!Common::flag_is_set($policy, self::WHERE_ONLY)) { 00928 $recent_values = $this->get_field_values(); 00929 foreach($recent_values as $column => $value) { 00930 if (is_null($value)) { 00931 $query->add_where($column, DBWhere::OP_IS_NULL); 00932 } 00933 else { 00934 $query->add_where($column, '=', $value); 00935 } 00936 } 00937 } 00938 00939 $query->set_limit($this->limit[0], $this->limit[1]); 00940 foreach($this->order_by as $order_by) { 00941 $query->add_order(key($order_by), current($order_by)); 00942 } 00943 00944 $use_distinct = false; 00945 foreach($this->joins as $join) { 00946 $joined_table = $join['table']; 00947 $conditions = $join['conditions']; 00948 $joined_query = $query->add_join($joined_table); 00949 $joined_query->set_join_type(Arr::get_item($join, 'type', DBQueryJoined::INNER)); 00950 if (count($conditions) > 0) { 00951 $joined_query->set_policy(DBQuery::NONE); 00952 foreach($conditions as $cond) { 00953 $joined_query->add_join_condition_object($cond); 00954 } 00955 } 00956 $use_distinct = $use_distinct || ($joined_query->get_relation_type() != DBRelation::ONE_TO_ONE); 00957 if ($joined_table instanceof DataObjectBase) { 00958 $joined_table->configure_select_query($joined_query, self::NORMAL); 00959 } 00960 } 00961 if ($use_distinct) { 00962 $query->set_policy($query->get_policy() | DBQuerySelect::DISTINCT); 00963 } 00964 }
DataObjectBase::configure_update_query | ( | $ | query, | |
$ | policy | |||
) | [protected] |
Configure an update query.
- Parameters:
-
DBQueryUpdate $query int $policy
Definition at line 1011 of file dataobjectbase.cls.php.
01011 { 01012 $fields = $this->get_field_values(); 01013 01014 $where = new DBWhereGroup($query->get_table()); 01015 $where->add_where_object($this->where); 01016 01017 if (!Common::flag_is_set($policy, self::WHERE_ONLY)) { 01018 foreach($this->get_table_keys() as $column => $field) { 01019 if (!isset($fields[$column])) { 01020 // Prevent unwanted mass updates... 01021 throw new Exception(tr('Trying to UPDATE without all keys set. If intended, use WHERE_ONLY policy', 'core')); 01022 } 01023 unset($fields[$column]); 01024 $where->add_where($column, '=', $this->$column); 01025 } 01026 } 01027 01028 $query->add_where_object($where); 01029 $query->set_fields($fields); 01030 }
DataObjectBase::count | ( | ) |
Count resulting items.
- Returns:
- int
Implements ISearchAdapter.
Definition at line 591 of file dataobjectbase.cls.php.
00591 { 00592 $query = $this->create_count_query(); 00593 $result = DB::query($query); 00594 $ret = false; 00595 if ($result->get_status()->is_ok()) { 00596 $arr = $result->fetch(); 00597 $ret = Arr::get_item($arr, 'c', 0); 00598 } 00599 return $ret; 00600 }
DataObjectBase::create_count_query | ( | $ | policy =
self::NORMAL |
) |
Prepare a count query.
- Parameters:
-
int $policy If set to DataObjectBase::WHERE_ONLY, current values are ignored
- Returns:
- DBQuerySelect
Definition at line 1102 of file dataobjectbase.cls.php.
01102 { 01103 $query = new DBQueryCount($this); 01104 $this->configure_count_query($query, self::NORMAL); 01105 $this->execute_query_hooks($query); 01106 return $query; 01107 }
DataObjectBase::create_delete_query | ( | $ | policy =
self::NORMAL |
) |
Prepare a delete query.
- Parameters:
-
int $policy If set to DataObjectBase::WHERE_ONLY, current values are ignored
- Returns:
- DBQueryDelete
Definition at line 1060 of file dataobjectbase.cls.php.
01060 { 01061 $query = new DBQueryDelete($this); 01062 $this->configure_delete_query($query, $policy); 01063 $this->execute_query_hooks($query); 01064 return $query; 01065 }
DataObjectBase::create_insert_query | ( | ) |
Prepare an insert query.
- Returns:
- DBQueryInsert
Definition at line 971 of file dataobjectbase.cls.php.
00971 { 00972 $query = new DBQueryInsert($this); 00973 $this->configure_insert_query($query); 00974 $this->execute_query_hooks($query); 00975 return $query; 00976 }
DataObjectBase::create_replace_query | ( | ) |
Prepare an insert query.
- Returns:
- DBQueryInsert
Definition at line 1037 of file dataobjectbase.cls.php.
01037 { 01038 $query = new DBQueryReplace($this); 01039 $this->configure_replace_query($query); 01040 $this->execute_query_hooks($query); 01041 return $query; 01042 }
DataObjectBase::create_select_query | ( | $ | policy =
self::NORMAL |
) |
Prepare a select query.
- Parameters:
-
int $policy If set to DataObjectBase::WHERE_ONLY, current values are ignored
- Returns:
- DBQuerySelect
Definition at line 911 of file dataobjectbase.cls.php.
00911 { 00912 $query = new DBQuerySelect($this, DBQuerySelect::NONE); 00913 $this->configure_select_query($query, $policy); 00914 $this->execute_query_hooks($query); 00915 return $query; 00916 }
DataObjectBase::create_table_object | ( | ) | [protected] |
Create the table object describing this dataobejcts table.
Reimplemented in DAOCache, DAOFormvalidations, DAOSessions, DAOCoursesTest, DAORoomsTest, DAOStudentsTest, DAOStudentsTest2CoursesTest, DAOTeachersTest, and DAOSystemupdates.
Definition at line 1158 of file dataobjectbase.cls.php.
01158 { 01159 return new DBTable('test'); 01160 }
DataObjectBase::create_update_query | ( | $ | policy =
self::NORMAL |
) |
Prepare an update query.
- Parameters:
-
int $policy If set to DataObjectBase::WHERE_ONLY, current values are ignored
- Returns:
- DBQueryUpdate
Definition at line 998 of file dataobjectbase.cls.php.
00998 { 00999 $query = new DBQueryUpdate($this); 01000 $this->configure_update_query($query, $policy); 01001 $this->execute_query_hooks($query); 01002 return $query; 01003 }
DataObjectBase::delete | ( | $ | policy =
self::NORMAL |
) |
Delete this object.
- Parameters:
-
int $policy If DBDataObject::WHERE_ONLY is used, no conditions are build automatically
- Returns:
- Status
Implements IDataObject.
Definition at line 290 of file dataobjectbase.cls.php.
00290 { 00291 $query = $this->create_delete_query($policy); 00292 return DB::execute($query->get_sql(), $query->get_table()->get_table_driver()); 00293 }
DataObjectBase::equals | ( | $ | other | ) |
Returns true if a both this and the given object are identical.
The codes tests if table and all fields are identical. Note that this function may return false in cases when is_same_as() returns true.
- Parameters:
-
IDataObject $other
- Returns:
- bool
Implements IDataObject.
Definition at line 571 of file dataobjectbase.cls.php.
00571 { 00572 $ret = ($other instanceof IDataObject); 00573 $ret = $ret && ($this->get_table_name() === $other->get_table_name()); 00574 if ($ret) { 00575 foreach($this->get_table_fields() as $name => $field_object) { 00576 $ret = $ret && ($this->$name === $other->$name); 00577 } 00578 } 00579 return $ret; 00580 }
DataObjectBase::execute | ( | ) |
Implemented from ISearchAdapter.
Synomy to find_array()
This function behaves like find_array(), it may however be overloaded to behave differently!
Implements ISearchAdapter.
Definition at line 615 of file dataobjectbase.cls.php.
00615 { 00616 return $this->find_array(); 00617 }
DataObjectBase::execute_query_hooks | ( | $ | query | ) | [protected] |
DataObjectBase::fetch | ( | ) |
fetches next row into this objects var's
returns true on success false on failure
Example $object = new mytable(); $object->name = "fred"; $object->find(); $store = array(); while ($object->fetch()) { echo $this->ID; $store[] = $object; // builds an array of object lines. }
- Returns:
- boolean True on success
Implements IDataObject.
Reimplemented in DataObjectCached.
Definition at line 442 of file dataobjectbase.cls.php.
00442 { 00443 $ret = false; 00444 if (!empty($this->resultset)) { 00445 $arr_data = $this->resultset->fetch(); 00446 if ($arr_data) { 00447 foreach($arr_data as $prop => $value) { 00448 $field = $this->get_table_field($prop); 00449 if ($field) { 00450 $value = $field->convert_result($value); 00451 } 00452 $this->$prop = $value; 00453 } 00454 $ret = true; 00455 } 00456 else { 00457 $this->resultset = null; 00458 } 00459 } 00460 return $ret; 00461 }
DataObjectBase::fetch_array | ( | ) |
Fetch and return an array.
- Returns:
- array Array of IDataObject
Implements IDataObject.
Definition at line 468 of file dataobjectbase.cls.php.
00468 { 00469 $arrRet = array(); 00470 while ($this->fetch()) { 00471 $arrRet[] = clone($this); 00472 } 00473 00474 return $arrRet; 00475 }
DataObjectBase::find | ( | $ | policy =
self::NORMAL |
) |
Find results, either normal or crosstable.
for example
$object = new mytable(); $object->ID = 1; $object->find();
- Parameters:
-
int $policy If set to DataObejctBase::AUTOFETCH, first record is fetched automatically
- Returns:
- int Number of rows found
Implements IDataObject.
Definition at line 410 of file dataobjectbase.cls.php.
00410 { 00411 $query = $this->create_select_query(); 00412 return $this->query($query->get_sql(), $policy); 00413 }
DataObjectBase::find_action_command | ( | $ | name | ) | [protected] |
Resolve action name to command.
Optionally, params can be added in brackets like 'status[DISABLED]'
- Parameters:
-
string $name
- Returns:
- ICommand Command, if any, false otherwise
Definition at line 889 of file dataobjectbase.cls.php.
00889 { 00890 $params = false; 00891 $pos_params = strpos($name, '['); 00892 if ($pos_params !== false) { 00893 $params = trim(substr($name, $pos_params + 1), ']'); 00894 $name = substr($name, 0, $pos_params); 00895 $params = explode(',', $params); 00896 } 00897 $ret = CommandsFactory::create_command($this, $name, $params); 00898 return $ret; 00899 }
DataObjectBase::find_array | ( | ) |
Return array of elements, based on current configuration.
- Returns:
- array Array of IDataObject
Implements IDataObject.
Definition at line 420 of file dataobjectbase.cls.php.
00420 { 00421 $this->find(); 00422 return $this->fetch_array(); 00423 }
DataObjectBase::get | ( | $ | column_or_value, | |
$ | value = null |
|||
) |
Finds and fetches first result of a select for $value on $column_or_value.
If $value is empty, this function assumes $column_or_value contains the value of first key column
- Parameters:
-
mixed $column_or_value mixed $value
- Returns:
- Bool. True on success, false, if no record was found
Implements IDataObject.
Definition at line 380 of file dataobjectbase.cls.php.
00380 { 00381 if (empty($value)) { 00382 $value = $column_or_value; 00383 $keys = array_keys($this->get_table_keys()); 00384 $column_or_value = Arr::get_item($keys, 0, false); 00385 } 00386 if (empty($column_or_value)) { 00387 throw new Exception(tr('No column set for get', 'core')); 00388 } 00389 00390 $query = new DBQuerySelect($this); 00391 $query->add_where($column_or_value, '=', $value); 00392 $query->set_limit(1); 00393 00394 $this->resultset = DB::query($query->get_sql(), $query->get_table()->get_table_driver()); 00395 return $this->fetch(); 00396 }
DataObjectBase::get_action_source_name | ( | ) |
Identify for generic actionh processing.
- Returns:
- string
Implements IActionSource.
Definition at line 861 of file dataobjectbase.cls.php.
00861 { 00862 return $this->get_table_name(); 00863 }
DataObjectBase::get_actions | ( | $ | user, | |
$ | context = 'view' , |
|||
$ | params = false |
|||
) |
Get all actions.
This function sends first calls get_actions_for_context() on this instance and afterwards invokes an event "get_actions". Clients may overload, extend or delete actions by returning an array with according key.
Actions may be passed in two ways. Either as an instance of IAction (which also includes commands) or as a string. In this case the array key is the action (or command) name, the value is the description that will get displayed. For Commands, however, the command's description may be used.
Optionally, params can be added in brackets like 'status[DISABLED]' => 'Disable this item'.
All actions will get access checked, and removed if access is denied
- Parameters:
-
mixed "Access Request Object", e.g. a user String The context. Some actions may not be approbiate in some situations. For example, action 'edit' should not be returned when editing. This can be expressed through a context named 'edit'. Default context is 'view'. mixed Any params
- Returns:
- Array Array of IAction instances
Implements IActionSource.
Definition at line 804 of file dataobjectbase.cls.php.
00804 { 00805 $actions = $this->get_actions_for_context($context, $user, $params); 00806 // Raise event to collect more actions 00807 $actions_event_result = array(); 00808 $actions_event_params = array( 00809 'source' => $this, 00810 'source_name' => $this->get_action_source_name(), 00811 'context' => $context, 00812 'params' => $params, 00813 'instance_actions' => $actions 00814 ); 00815 EventSource::Instance()->invoke_event('get_actions', $actions_event_params, $actions_event_result); 00816 $actions = array_merge($actions, $actions_event_result); 00817 00818 // Delete current action 00819 unset($actions[$context]); 00820 $ret = array(); 00821 foreach($actions as $name => $iaction_or_description) { 00822 if ($iaction_or_description instanceof IAction) { 00823 $ret[] = $iaction_or_description; 00824 } 00825 else { 00826 $description = $iaction_or_description; 00827 if (empty($description)) { 00828 continue; 00829 } 00830 $actionsource = $this; 00831 $action = $name; 00832 if (strpos($name, '::') !== false) { 00833 $tmp = explode('::', $name); 00834 $actionsource = array_shift($tmp); 00835 $action = implode('_', $tmp); 00836 $name = str_replace('::', '_', $name); 00837 } 00838 00839 $cmd = $this->find_action_command($name); 00840 if ($cmd) { 00841 if ($cmd->can_execute($user)) { 00842 $ret[] = $cmd; 00843 } 00844 } 00845 else { 00846 if (AccessControl::is_allowed($action, $actionsource, $params, $user)) { 00847 $ret[] = new ActionBase($this, $name, $description); 00848 } 00849 } 00850 } 00851 } 00852 00853 return $ret; 00854 }
DataObjectBase::get_actions_for_context | ( | $ | context, | |
$ | user, | |||
$ | params | |||
) | [protected] |
To be overloaded.
Returns array of actions with action title as key and action description as value
Subclasses can return array of actions, this class will detect if they are commands or actions.
Optionally, params can be added in brackets like 'status[DISABLED]' => 'Disable this item'.
- Parameters:
-
string $context mixed $user mixed $params
- Returns:
- array
Definition at line 877 of file dataobjectbase.cls.php.
DataObjectBase::get_field_values | ( | $ | only_set = true |
) | [protected] |
Returns aoociative array of fields and values.
Definition at line 188 of file dataobjectbase.cls.php.
00188 { 00189 $b_all = !$only_set; 00190 $ret = array(); 00191 foreach($this->get_table_fields() as $column => $field) { 00192 if ($b_all || isset($this->$column)) { 00193 $v = $this->$column; 00194 if ($v instanceof DBNull) { 00195 $v = NULL; 00196 } 00197 $ret[$column] = $v; 00198 } 00199 } 00200 return $ret; 00201 }
DataObjectBase::get_filters | ( | ) |
Return array of filters.
Array has filter as key and a readable description as value
Implements ISearchAdapter.
Definition at line 651 of file dataobjectbase.cls.php.
DataObjectBase::get_matching_relations | ( | IDBTable $ | other | ) |
Returns relations between two tables.
- Parameters:
-
IDBTable $other
- Returns:
- array Array of IDBRelations
Implements IDBTable.
Definition at line 756 of file dataobjectbase.cls.php.
DataObjectBase::get_properties_to_exclude_for_sleep | ( | ) | [protected] |
Definition at line 93 of file dataobjectbase.cls.php.
DataObjectBase::get_sort_default_column | ( | ) |
Get the column to sort by default.
Implements ISearchAdapter.
Definition at line 629 of file dataobjectbase.cls.php.
DataObjectBase::get_sortable_columns | ( | ) |
Return array of sortable columns.
Array has column name as key and some sort of sort-column-object or an array as values
Implements ISearchAdapter.
Definition at line 622 of file dataobjectbase.cls.php.
DataObjectBase::get_table_alias | ( | ) |
Returns alias of table, if any.
- Returns:
- string
Implements IDBTable.
Definition at line 691 of file dataobjectbase.cls.php.
DataObjectBase::get_table_alias_escaped | ( | ) |
Returns alias of table, if any - but escaped.
- Returns:
- string
Implements IDBTable.
Definition at line 709 of file dataobjectbase.cls.php.
DataObjectBase::get_table_constraints | ( | ) |
Returns array of constraints.
- Returns:
- array Array with IDBConstraint instance as value
Implements IDBTable.
Definition at line 765 of file dataobjectbase.cls.php.
DataObjectBase::get_table_driver | ( | ) |
Returns DB driver fro this table.
- Returns:
- IDBDriver
Implements IDBTable.
Reimplemented in DAOSystemupdates.
Definition at line 774 of file dataobjectbase.cls.php.
DataObjectBase::get_table_field | ( | $ | column | ) |
Returns field fpr given column.
- Parameters:
-
string $column Column name
- Returns:
- IDBField Either field or false if no such field exists
Implements IDBTable.
Definition at line 728 of file dataobjectbase.cls.php.
DataObjectBase::get_table_fields | ( | ) |
Returns array of columns.
- Returns:
- array Associative array with column name as key and IDBField instance as value
Implements IDBTable.
Definition at line 718 of file dataobjectbase.cls.php.
DataObjectBase::get_table_keys | ( | ) |
Returns array of keys.
- Returns:
- array Associative array with column name as key and IDField instance as value
Implements IDBTable.
Definition at line 737 of file dataobjectbase.cls.php.
DataObjectBase::get_table_name | ( | ) |
Returns name of table.
- Returns:
- string
Implements IDBTable.
Definition at line 682 of file dataobjectbase.cls.php.
DataObjectBase::get_table_name_escaped | ( | ) |
Returns name of table, but escaped.
- Returns:
- string
Implements IDBTable.
Reimplemented in DAOSystemupdates.
Definition at line 700 of file dataobjectbase.cls.php.
DataObjectBase::get_table_relations | ( | ) |
Returns array of relations.
- Returns:
- array Array with IDBRelation instance as value
Implements IDBTable.
Definition at line 746 of file dataobjectbase.cls.php.
DataObjectBase::get_wheres | ( | ) |
Returns root collection of wheres.
- Returns:
- DBWhereGroup
Implements IDBWhereHolder.
Definition at line 513 of file dataobjectbase.cls.php.
DataObjectBase::init_table_object | ( | ) | [protected] |
Init table (create, retrieve) DBTable instance.
- Returns:
- IDBTable
Definition at line 55 of file dataobjectbase.cls.php.
00055 { 00056 // we assume we are named 'DAOtablename' 00057 $name = strtolower(get_class($this)); 00058 if (substr($name, 0, 3) == 'dao') { 00059 $name = substr($name, 3); 00060 } 00061 $ret = DBTableRepository::get($name); 00062 if (empty($ret)) { 00063 $ret = $this->create_table_object(); 00064 DBTableRepository::register($ret, $name); 00065 } 00066 return $ret; 00067 }
DataObjectBase::insert | ( | ) |
Insert data.
Autoincrement IDs will be automatically set.
- Returns:
- Status
Implements IDataObject.
Reimplemented in DataObjectTimestampedCached.
Definition at line 161 of file dataobjectbase.cls.php.
00161 { 00162 $query = $this->create_insert_query(); 00163 $connection = $query->get_table()->get_table_driver(); 00164 $ret = DB::execute($query->get_sql(), $connection); 00165 // find insert id 00166 $this->set_last_insert_id($connection); 00167 return $ret; 00168 }
DataObjectBase::is_same_as | ( | $ | other | ) |
Returns true if a both this and the given object refer to the same instance in the DB.
The codes tests if table and key fields are identical. Note that this function returns true even if some properties are not identical. Use equals() to test this
- Parameters:
-
IDataObject $other
- Returns:
- bool
Implements IDataObject.
Definition at line 551 of file dataobjectbase.cls.php.
00551 { 00552 $ret = ($other instanceof IDataObject); 00553 $ret = $ret && ($this->get_table_name() === $other->get_table_name()); 00554 if ($ret) { 00555 foreach($this->get_table_keys() as $name => $field_object) { 00556 $ret = $ret && ($this->$name === $other->$name); 00557 } 00558 } 00559 return $ret; 00560 }
DataObjectBase::join | ( | IDBTable $ | other, | |
$ | conditions =
array() , |
|||
$ | type = DBQueryJoined::INNER |
|||
) |
Join another table to this.
- Parameters:
-
IDBTable $other array $conditions Array of DBJoinCondition. When ommited Gyro will figure out join conditions by itself string $type One of DBQueryJoined::INNER, DBQueryJoined::LEFT, or DBQueryJoined::RIGHT
Definition at line 524 of file dataobjectbase.cls.php.
00524 { 00525 $this->joins[] = array( 00526 'table' => $other, 00527 'conditions' => Arr::force($conditions, false), 00528 'type' => $type 00529 ); 00530 }
DataObjectBase::limit | ( | $ | start = 0 , |
|
$ | number_of_items =
0 |
|||
) |
DataObjectBase::query | ( | $ | query, | |
$ | policy =
self::NORMAL , |
|||
$ | connection = false |
|||
) |
Execute a query (SELECT).
Similar to find, but excepts any string.
Do not use with INSERT, UPDATE, or similar. This can be executed using DB::execute()
- Parameters:
-
string $query int $policy If set to DataObejctBase::AUTOFETCH, first record is fetched automatically
- Returns:
- int Number of rows found
Definition at line 1130 of file dataobjectbase.cls.php.
01130 { 01131 $connection = ($connection) ? $connection : $this->get_table_driver(); 01132 $this->resultset = DB::query($query, $connection); 01133 $ret = $this->resultset->get_row_count(); 01134 01135 if (Common::flag_is_set($policy, self::AUTOFETCH)) { 01136 $this->fetch(); 01137 } 01138 01139 return $ret; 01140 }
DataObjectBase::quote | ( | $ | val | ) |
Quote and escape a string.
- Parameters:
-
string $val
- Returns:
- string
Implements IDataObject.
Definition at line 538 of file dataobjectbase.cls.php.
00538 { 00539 return DB::quote($val, $this->get_table_driver()); 00540 }
DataObjectBase::read_from_array | ( | $ | values | ) |
read properties of object form array
- Parameters:
-
array $values Associative array with property name as key and property value as value
Implements IDataObject.
Definition at line 300 of file dataobjectbase.cls.php.
00300 { 00301 foreach($this->get_table_fields() as $prop => $field) { 00302 $value = $field->read_from_array($values); 00303 if (!is_null($value)) { 00304 $this->$prop = $value; 00305 } 00306 } 00307 }
DataObjectBase::register_query_hook | ( | IDataObjectQueryHook $ | hook | ) |
Register hook to be called for every query created.
- Parameters:
-
IDataObjectQueryHook $hook
Definition at line 1147 of file dataobjectbase.cls.php.
DataObjectBase::replace | ( | ) |
Inserts if data does not exist, updates else.
This function queries the database, that is it does not use MySQL "replace into" It does not handle autoupdated ids. That is: It only works if all keys are set
- Returns:
- Status
Implements IDataObject.
Definition at line 211 of file dataobjectbase.cls.php.
00211 { 00212 $arr_keys = $this->get_table_keys(); 00213 $b_keys_complete = true; 00214 foreach($arr_keys as $key_column => $field) { 00215 // All keys empty => Insert 00216 if (empty($this->$key_column)) { 00217 $b_keys_complete = false; 00218 break; 00219 } 00220 } 00221 00222 // Only run if all keys are set 00223 if (!$b_keys_complete) { 00224 return new Status(tr( 00225 'Replace cannot be called with a key beeing empty', 00226 'core' 00227 )); 00228 } 00229 00230 $ret = new Status(); 00231 $driver = $this->get_table_driver(); 00232 if ($driver->has_feature(IDBDriver::FEATURE_REPLACE)) { 00233 $query = $this->create_replace_query(); 00234 $ret->merge(DB::execute($query, $driver)); 00235 } 00236 else { 00237 $ret->merge($this->replace_manual()); 00238 } 00239 return $ret; 00240 }
DataObjectBase::replace_manual | ( | ) | [protected] |
Inserts if data does not exist, updates else.
This function queries the database, that is it does not use MySQL "replace into" It does not handle autoupdated ids. That is: It only works if all keys are set
- Returns:
- Status
Definition at line 250 of file dataobjectbase.cls.php.
00250 { 00251 $arr_keys = $this->get_table_keys(); 00252 00253 // Retrieve old value 00254 $query = new DBQuerySelect($this, DBQuerySelect::FOR_UPDATE); 00255 foreach($arr_keys as $key_column => $field) { 00256 $query->add_where($key_column, '=', $this->$key_column); 00257 } 00258 $result = DB::query($query->get_sql(), $query->get_table()->get_table_driver()); 00259 00260 $ret = $result->get_status(); 00261 if ($ret->is_ok()) { 00262 if ($result->get_row_count() > 0) { 00263 $ret->merge($this->update()); 00264 } 00265 else { 00266 $ret->merge($this->insert()); 00267 } 00268 } 00269 return $ret; 00270 }
DataObjectBase::reset | ( | ) | [protected] |
Definition at line 1162 of file dataobjectbase.cls.php.
DataObjectBase::save | ( | ) |
Either update or insert object.
This function handles autoupdating IDs
- Returns:
- Status
Implements IDataObject.
Definition at line 143 of file dataobjectbase.cls.php.
00143 { 00144 $bInsert = true; 00145 foreach($this->get_table_keys() as $column => $field) { 00146 $bInsert = $bInsert && empty($this->$column); 00147 } 00148 if ($bInsert) { 00149 return $this->insert(); 00150 } 00151 else { 00152 return $this->replace(); 00153 } 00154 }
DataObjectBase::set_default_values | ( | ) |
Sets all default values on instance.
Previous values are overwritten!
Implements IDataObject.
Definition at line 130 of file dataobjectbase.cls.php.
00130 { 00131 foreach($this->get_table_fields() as $column => $field) { 00132 $this->$column = $field->get_field_default(); 00133 } 00134 }
DataObjectBase::set_last_insert_id | ( | $ | connection | ) | [protected] |
Set the last insert id if there is an autoincrement INTEGER field.
Definition at line 173 of file dataobjectbase.cls.php.
00173 { 00174 // find insert id 00175 $table_keys = $this->get_table_keys(); 00176 $id_field = array_shift($table_keys); 00177 if ($id_field && $id_field instanceof DBFieldInt && $id_field->has_policy(DBFieldInt::AUTOINCREMENT)) { 00178 $fieldname = $id_field->get_field_name(); 00179 if (empty($this->$fieldname)) { 00180 $this->$fieldname = DB::last_insert_id($connection); 00181 } 00182 } 00183 }
DataObjectBase::set_table_alias | ( | $ | alias | ) |
Set a table alias for this instance.
Definition at line 669 of file dataobjectbase.cls.php.
00669 { 00670 $this->table = new DBTableAlias($this->table, $alias); 00671 }
DataObjectBase::sort | ( | $ | column, | |
$ | order = self::ASC |
|||
) |
Sort result by colum.
- Parameters:
-
String column name Enum 'asc' or 'desc'
Implements ISearchAdapter.
Definition at line 639 of file dataobjectbase.cls.php.
DataObjectBase::to_string | ( | ) |
Definition at line 106 of file dataobjectbase.cls.php.
00106 { 00107 $ret = ''; 00108 $fields = array(); 00109 foreach($this->get_table_keys() as $key_field) { 00110 /* @var $key_field DBField */ 00111 $col_name = $key_field->get_field_name(); 00112 $fields[$col_name] = $this->$col_name; 00113 } 00114 00115 $ret .= $this->get_table_name(); 00116 if (count($fields)) { 00117 $ret .= '[' . Arr::implode('|', $fields, '=') . ']'; 00118 } 00119 00120 return $ret; 00121 }
DataObjectBase::unset_internals | ( | $ | arr_properties | ) |
Removes all properties from array that are marked using DBField::INTERNAL.
- Parameters:
-
array $arr_properties Associative array with field name as key
- Returns:
- array The cleaned array
Definition at line 315 of file dataobjectbase.cls.php.
00315 { 00316 $ret = array(); 00317 // Copy only elements that are not INTERNAL 00318 foreach($arr_properties as $field => $value) { 00319 $dbfield = $this->get_table_field($field); 00320 if ($dbfield && $dbfield->has_policy(DBField::INTERNAL)) { 00321 continue; 00322 } 00323 $ret[$field] = $value; 00324 } 00325 // Remove primary keys from cleaned array 00326 foreach($this->get_table_keys() as $field => $tmp) { 00327 unset($ret[$field]); 00328 } 00329 return $ret; 00330 }
DataObjectBase::update | ( | $ | policy =
self::NORMAL |
) |
Update current item.
- Parameters:
-
int $policy If DBDataObject::WHERE_ONLY is used, no conditions are build automatically
- Returns:
- Status
Implements IDataObject.
Reimplemented in DataObjectTimestampedCached.
Definition at line 278 of file dataobjectbase.cls.php.
00278 { 00279 $query = $this->create_update_query($policy); 00280 return DB::execute($query->get_sql(), $query->get_table()->get_table_driver()); 00281 }
DataObjectBase::validate | ( | ) |
Validate this object.
- Returns:
- Status Error
Implements IDataObject.
Definition at line 337 of file dataobjectbase.cls.php.
00337 { 00338 $ret = new Status(); 00339 $fields = $this->get_field_values(false); 00340 foreach($this->get_table_fields() as $column => $field) { 00341 $val = Arr::get_item($fields, $column, null); 00342 $ret->merge($field->validate($val)); 00343 } 00344 if ($ret->is_ok()) { 00345 // Validate relations 00346 foreach($this->get_table_relations() as $relation) { 00347 /* @var $relation IDBRelation */ 00348 $arr_val = array(); 00349 foreach($relation->get_fields() as $column => $fieldrelation) { 00350 // TODO refactor this 00351 $arr_val[$column] = Arr::get_item($fields, $column, null); 00352 } 00353 $ret->merge($relation->validate($arr_val)); 00354 } 00355 foreach($this->get_table_constraints() as $constraint) { 00356 /* @var $constraint IDBConstraint */ 00357 $arr_val = array(); 00358 $arr_keys = array(); 00359 foreach($constraint->get_fields() as $column) { 00360 $arr_val[$column] = Arr::get_item($fields, $column, null); 00361 } 00362 foreach($this->get_table_keys() as $column => $field_object) { 00363 $arr_keys[$column] = Arr::get_item($fields, $column, null); 00364 } 00365 $ret->merge($constraint->validate($arr_val, $arr_keys)); 00366 } 00367 } 00368 return $ret; 00369 }
Member Data Documentation
DataObjectBase::$joins = array()
[protected] |
Definition at line 41 of file dataobjectbase.cls.php.
DataObjectBase::$limit = array(0,0)
[protected] |
Definition at line 34 of file dataobjectbase.cls.php.
DataObjectBase::$order_by = array()
[protected] |
Definition at line 35 of file dataobjectbase.cls.php.
DataObjectBase::$queryhooks = array()
[protected] |
Definition at line 42 of file dataobjectbase.cls.php.
DataObjectBase::$resultset = null
[protected] |
Definition at line 22 of file dataobjectbase.cls.php.
DataObjectBase::$table = null
[protected] |
Definition at line 16 of file dataobjectbase.cls.php.
DataObjectBase::$where = null
[protected] |
Definition at line 28 of file dataobjectbase.cls.php.
The documentation for this class was generated from the following file:
- gyro/core/model/base/dataobjectbase.cls.php