DBRelation Class Reference
[Model]
Defines a relation between two tables. More...
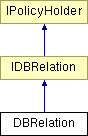
Public Member Functions |
|
__construct ($target_table, $fields=null, $policy=self::NOT_NULL, $type=self::ONE_TO_MANY) | |
add_field_relation (IDBFieldRelation $field) | |
get_fields () | |
Returns field taking parts in this relation.
|
|
get_null_allowed () | |
Returns true, if null values are allowed.
|
|
get_policy () | |
Return policy. |
|
get_reversed_fields () | |
Returns array of fields, but fields get
reversed before. |
|
get_target_table_name () | |
Return target table name. |
|
get_type () | |
Returns type of relation. |
|
has_policy ($policy) | |
Returns true, if client has given policy.
|
|
set_policy ($policy) | |
Set policy. |
|
validate ($arr_fields) | |
Check if relation conditions are fullfiled.
|
|
Public Attributes |
|
const | MANY_TO_MANY = 3 |
const | NOT_NULL = 1 |
Do not allow source field(s) to be null.
|
|
const | ONE_TO_MANY = 2 |
const | ONE_TO_ONE = 1 |
Protected Attributes |
|
$arr_fields = array() | |
$policy | |
$target_table | |
$type |
Detailed Description
Defines a relation between two tables.
Definition at line 8 of file dbrelation.cls.php.
Constructor & Destructor Documentation
DBRelation::__construct | ( | $ | target_table, | |
$ | fields = null , |
|||
$ | policy =
self::NOT_NULL , |
|||
$ | type =
self::ONE_TO_MANY |
|||
) |
Definition at line 23 of file dbrelation.cls.php.
00023 { 00024 $this->target_table = $target_table; 00025 $this->policy = $policy; 00026 $this->type = $type; 00027 00028 if (!empty($fields)) { 00029 foreach(Arr::force($fields) as $field) { 00030 $this->add_field_relation($field); 00031 } 00032 } 00033 }
Member Function Documentation
DBRelation::add_field_relation | ( | IDBFieldRelation $ | field | ) |
Definition at line 124 of file dbrelation.cls.php.
DBRelation::get_fields | ( | ) |
Returns field taking parts in this relation.
- Returns:
- array Associative array with column name as key and IDFieldRelation instance as value
Implements IDBRelation.
Definition at line 49 of file dbrelation.cls.php.
DBRelation::get_null_allowed | ( | ) |
Returns true, if null values are allowed.
- Returns:
- bool
Implements IDBRelation.
Definition at line 71 of file dbrelation.cls.php.
00071 { 00072 return !Common::flag_is_set($this->policy, self::NOT_NULL); 00073 }
DBRelation::get_policy | ( | ) |
Return policy.
- Returns:
- int
Implements IPolicyHolder.
Definition at line 133 of file dbrelation.cls.php.
DBRelation::get_reversed_fields | ( | ) |
Returns array of fields, but fields get reversed before.
- Returns:
- array Associative array with column name as key and IDFieldRelation instance as value
Implements IDBRelation.
Definition at line 58 of file dbrelation.cls.php.
00058 { 00059 $ret = array(); 00060 foreach($this->get_fields() as $key => $field) { 00061 $ret[$field->get_target_field_name()] = $field->reverse(); 00062 } 00063 return $ret; 00064 }
DBRelation::get_target_table_name | ( | ) |
Return target table name.
- Returns:
- string
Implements IDBRelation.
Definition at line 40 of file dbrelation.cls.php.
DBRelation::get_type | ( | ) |
Returns type of relation.
- Returns:
- int
Implements IDBRelation.
Definition at line 161 of file dbrelation.cls.php.
DBRelation::has_policy | ( | $ | policy | ) |
Returns true, if client has given policy.
- Parameters:
-
int $policy
- Returns:
- bool
Implements IPolicyHolder.
Definition at line 152 of file dbrelation.cls.php.
00152 { 00153 return Common::flag_is_set($this->policy, $policy); 00154 }
DBRelation::set_policy | ( | $ | policy | ) |
Set policy.
- Parameters:
-
int $policy
Implements IPolicyHolder.
Definition at line 142 of file dbrelation.cls.php.
00142 { 00143 $this->policy = $policy; 00144 }
DBRelation::validate | ( | $ | arr_fields | ) |
Check if relation conditions are fullfiled.
This checks from a source perspective, that is:
- See if source field conditions are met (e.g. NOT NULL)
- Check if there is a least one record on target table
- Parameters:
-
array Associative array of form fieldname => fieldvalue
- Returns:
- Status
Implements IDBRelation.
Definition at line 84 of file dbrelation.cls.php.
00084 { 00085 $ret = new Status(); 00086 // Create target instance 00087 $dao = DB::create($this->target_table); 00088 00089 $b_all_fields_null = true; 00090 foreach($this->get_fields() as $column => $relation) { 00091 // Check each fieldrelation 00092 $value = Arr::get_item($arr_fields, $column, null); 00093 $b_all_fields_null = $b_all_fields_null && is_null($value); 00094 00095 // Set on target class 00096 $target_field = $relation->get_target_field_name(); 00097 $dao->$target_field = $value; 00098 } 00099 // No item on target table specified 00100 if ($b_all_fields_null) { 00101 if (!$this->get_null_allowed()) { 00102 $ret->append(tr( 00103 'No instance set for relation to table %target', 00104 'core', 00105 array( 00106 '%target' => tr($this->get_target_table_name(), 'global') 00107 ) 00108 )); 00109 } 00110 } 00111 else if ($dao->count() == 0) { 00112 // No such target instance exists 00113 $ret->append(tr( 00114 'No matching instance found for relation to table %target', 00115 'core', 00116 array( 00117 '%target' => tr($this->get_target_table_name(), 'global') 00118 ) 00119 )); 00120 } 00121 return $ret; 00122 }
Member Data Documentation
DBRelation::$arr_fields = array()
[protected] |
Definition at line 19 of file dbrelation.cls.php.
DBRelation::$policy
[protected] |
Definition at line 20 of file dbrelation.cls.php.
DBRelation::$target_table
[protected] |
Definition at line 18 of file dbrelation.cls.php.
DBRelation::$type [protected] |
Definition at line 21 of file dbrelation.cls.php.
const DBRelation::MANY_TO_MANY = 3 |
Definition at line 16 of file dbrelation.cls.php.
const DBRelation::NOT_NULL = 1 |
Do not allow source field(s) to be null.
Definition at line 12 of file dbrelation.cls.php.
const DBRelation::ONE_TO_MANY = 2 |
Definition at line 15 of file dbrelation.cls.php.
const DBRelation::ONE_TO_ONE = 1 |
Definition at line 14 of file dbrelation.cls.php.
The documentation for this class was generated from the following file:
- gyro/core/model/base/dbrelation.cls.php