RouteBase Class Reference
[Controller]
Basic route, which handles stub urls. More...
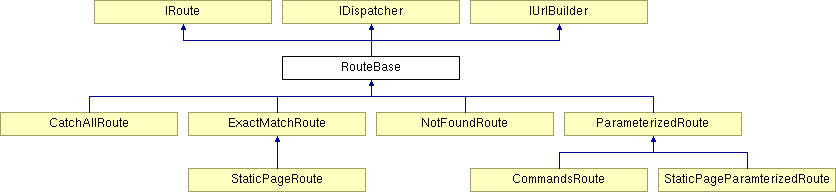
Public Member Functions |
|
__construct ($path, $controller, $action, $decorators=null) | |
Contructor. |
|
append_renderdecorator (IRenderDecorator $dec) | |
Append a RenderDecorator to the list of
render decorators. |
|
build_url ($absolute_or_relative, $params=null) | |
Build the URL. |
|
get_renderer ($page_data) | |
Returns a suitable renderer. |
|
identify () | |
Return a string that identifies this Route -
e.g for debug purposes. |
|
initialize ($page_data) | |
Initialize the data passed. |
|
invoke ($page_data) | |
Invoke the action on controller. |
|
is_directory () | |
Returns true, if this route is a directory
(that is: ends with '/'). |
|
prepend_renderdecorator (IRenderDecorator $dec) | |
Prepend a RenderDecorator to the list of
render decorators. |
|
weight_against_path ($path) | |
Weight this token against path. |
|
Static Public Member Functions |
|
static | split_route_id ($route_id) |
Split a route identifier back into
controller and action. |
|
Protected Member Functions |
|
build_url_base ($absolute_or_relative) | |
build_url_path ($params) | |
Build the URL (except base part). |
|
check_action_func ($controller, $funcname) | |
Cehck if given action exists on controller.
|
|
get_action_func_name ($action) | |
Return fucntion to invoke for given action.
|
|
get_default_render_decorators () | |
Returns array of renderdecorators to be
append to decortators passed by controller. |
|
initialize_adjust_path ($page_data) | |
Adjust path during initializationh process.
|
|
initialize_cache_manager ($page_data) | |
Initialize the cache manager. |
|
invoke_action_func ($controller, $funcname, $page_data) | |
Invokes given action function on given
controller. |
|
Protected Attributes |
|
$action = '' | |
$controller = null | |
$decorators = null | |
$is_directory = false | |
$path = '' | |
$scheme = '' |
Detailed Description
Basic route, which handles stub urls.
By handling stubs, a route defined for example 'a/b' will match 'a/b/c', too (unless there isn't another routes that matches better). The according action shoulds check the PathStack of PageData for the remaining part of the URL.
Definition at line 17 of file routebase.cls.php.
Constructor & Destructor Documentation
RouteBase::__construct | ( | $ | path, | |
$ | controller, | |||
$ | action, | |||
$ | decorators = null |
|||
) |
Contructor.
- Parameters:
-
string $path The URL this route is responsible for IController $controller The controller to invoke string $action The function to invoke on controller mixed Array or single instance of IRouteDecorator
Reimplemented in CommandsRoute.
Definition at line 33 of file routebase.cls.php.
00033 { 00034 $this->controller = $controller; 00035 $this->action = $action; 00036 // check if path contains a protocol 00037 $pos_scheme = strpos($path, '://'); 00038 if ($pos_scheme !== false) { 00039 $this->scheme = substr($path, 0, $pos_scheme); 00040 $path = substr($path, $pos_scheme + 3); 00041 } else { 00042 $this->scheme = Config::get_value(Config::DEFAULT_SCHEME); 00043 } 00044 if ($this->scheme == 'https' && !Config::has_feature(Config::ENABLE_HTTPS)) { 00045 $this->scheme = 'http'; 00046 } 00047 $this->path = ($path !== '/') ? ltrim($path, '/') : $path; 00048 $this->is_directory = (substr($path, -1) === '/'); 00049 $this->decorators = is_array($decorators) ? $decorators : array($decorators); 00050 ActionMapper::register_url($action, $this); 00051 }
Member Function Documentation
RouteBase::append_renderdecorator | ( | IRenderDecorator $ | dec | ) |
Append a RenderDecorator to the list of render decorators.
- Parameters:
-
IRenderDecorator $dec
- Returns:
- void
Definition at line 107 of file routebase.cls.php.
RouteBase::build_url | ( | $ | absolute_or_relative, | |
$ | params = null |
|||
) |
Build the URL.
- Parameters:
-
bool $absolute_or_relative True to build an absolute URL, false to return path only mixed $params Further parameters to use to build URL
Implements IUrlBuilder.
Definition at line 285 of file routebase.cls.php.
00285 { 00286 $ret = $this->build_url_base($absolute_or_relative); 00287 $ret .= preg_replace('|/+|', '/', $this->build_url_path($params)); 00288 $ret = rtrim($ret, '.'); 00289 return $ret; 00290 }
RouteBase::build_url_base | ( | $ | absolute_or_relative | ) | [protected] |
Definition at line 312 of file routebase.cls.php.
00312 { 00313 if ($this->scheme != 'any' && Url::current()->get_scheme() != $this->scheme) { 00314 // E.g. a switch from http to https is required. Force absolute 00315 $absolute_or_relative = self::ABSOLUTE; 00316 } 00317 if (Url::current()->get_host() != Config::get_value(Config::URL_DOMAIN)) { 00318 $absolute_or_relative = self::ABSOLUTE; 00319 } 00320 $ret = Config::get_value(Config::URL_BASEDIR); 00321 if ($absolute_or_relative == self::ABSOLUTE) { 00322 switch ($this->scheme) { 00323 case 'https': 00324 $ret = Config::get_url(Config::URL_BASEURL_SAFE); 00325 break; 00326 case 'any': 00327 $ret = Url::create(Config::get_url(Config::URL_BASEURL))->set_scheme(Url::current()->get_scheme())->build(Url::ABSOLUTE); 00328 break; 00329 default: 00330 $ret = Config::get_url(Config::URL_BASEURL); 00331 break; 00332 } 00333 } 00334 return $ret; 00335 }
RouteBase::build_url_path | ( | $ | params | ) | [protected] |
Build the URL (except base part).
- Parameters:
-
mixed $params Further parameters to use to build URL
- Returns:
- string
Reimplemented in ExactMatchRoute, and ParameterizedRoute.
Definition at line 298 of file routebase.cls.php.
RouteBase::check_action_func | ( | $ | controller, | |
$ | funcname | |||
) | [protected] |
Cehck if given action exists on controller.
- Parameters:
-
object $controller The controller to invoke action on string $funcname Name of function
- Exceptions:
-
Exception if function does not exist on controller
Definition at line 257 of file routebase.cls.php.
00257 { 00258 if (method_exists($controller, $funcname) == false) { 00259 throw new Exception( 00260 tr('Action %a on controller %c not found', 'core', array('%a' => $funcname, '%c' => get_class($this->controller))) 00261 ); 00262 } 00263 }
RouteBase::get_action_func_name | ( | $ | action | ) | [protected] |
Return fucntion to invoke for given action.
- Parameters:
-
string Name of action
- Returns:
- string
Definition at line 271 of file routebase.cls.php.
00271 { 00272 return 'action_' . $action; 00273 }
RouteBase::get_default_render_decorators | ( | ) | [protected] |
Returns array of renderdecorators to be append to decortators passed by controller.
- Returns:
- array
Definition at line 116 of file routebase.cls.php.
00116 { 00117 return array( 00118 new DispatcherInvokeRenderDecorator($this) 00119 ); 00120 }
RouteBase::get_renderer | ( | $ | page_data | ) |
Returns a suitable renderer.
- Parameters:
-
PageData $page_data The page data
- Returns:
- IRenderer
Implements IRoute.
Definition at line 73 of file routebase.cls.php.
00073 { 00074 // Default render decorators 00075 $arr_default_decorator = $this->get_default_render_decorators(); 00076 $arr_overloaded_default_decorators = $page_data->get_render_decorators($this); 00077 $arr_decorators = array(); 00078 // Alllow cache managers as decorator 00079 foreach($this->decorators as $decorator) { 00080 if ($decorator instanceof ICacheManager) { 00081 $arr_decorators[] = new CacheRenderDecorator($decorator); 00082 } 00083 else { 00084 $arr_decorators[] = $decorator; 00085 } 00086 } 00087 $arr_decorators = array_merge($arr_decorators, $arr_overloaded_default_decorators, $arr_default_decorator); 00088 return new RendererChain($page_data, $arr_decorators); 00089 }
RouteBase::identify | ( | ) |
Return a string that identifies this Route - e.g for debug purposes.
Implements IRoute.
Reimplemented in NotFoundRoute.
Definition at line 125 of file routebase.cls.php.
RouteBase::initialize | ( | $ | page_data | ) |
Initialize the data passed.
- Parameters:
-
PageData $page_data
Implements IRoute.
Definition at line 146 of file routebase.cls.php.
00146 { 00147 if ($this->scheme != 'any' && Url::current()->get_scheme() != $this->scheme) { 00148 // redirect to given scheme 00149 Url::current()->set_scheme($this->scheme)->redirect(Url::PERMANENT); 00150 exit; 00151 } 00152 00153 $this->initialize_adjust_path($page_data); 00154 $this->initialize_cache_manager($page_data); 00155 }
RouteBase::initialize_adjust_path | ( | $ | page_data | ) | [protected] |
Adjust path during initializationh process.
- Parameters:
-
PageData $page_data
Reimplemented in ParameterizedRoute.
Definition at line 174 of file routebase.cls.php.
RouteBase::initialize_cache_manager | ( | $ | page_data | ) | [protected] |
Initialize the cache manager.
- Parameters:
-
PageData $page_data
Definition at line 162 of file routebase.cls.php.
RouteBase::invoke | ( | $ | page_data | ) |
Invoke the action on controller.
- Parameters:
-
PageData $page_data
- Returns:
- mixed Controller status
Implements IDispatcher.
Reimplemented in NotFoundRoute.
Definition at line 203 of file routebase.cls.php.
00203 { 00204 if (empty($this->controller)) { 00205 throw new Exception( 00206 tr('No controller on dispatcher %c', 'core', array('%c' => get_class($this))) 00207 ); 00208 } 00209 if (empty($this->action)) { 00210 throw new Exception( 00211 tr('No action on dispatcher %c', 'core', array('%c' => get_class($this))) 00212 ); 00213 } 00214 00215 // Check for action 00216 $funcname = $this->get_action_func_name($this->action); 00217 00218 // Invoke before-action (this is where the controller should do includes 00219 if (method_exists($this->controller, 'before_action')) { 00220 $this->controller->before_action(); 00221 } 00222 // Invoke action 00223 $status = $this->invoke_action_func($this->controller, $funcname, $page_data); 00224 if (empty($status) || $status == CONTROLLER_OK) { 00225 if ($page_data->get_pathstack()->current()) { 00226 // There still are items in the path, that is the URL couldn't be processed 00227 $status = CONTROLLER_NOT_FOUND; 00228 } 00229 else { 00230 $status = CONTROLLER_OK; 00231 } 00232 } 00233 $page_data->status_code = $status; 00234 }
RouteBase::invoke_action_func | ( | $ | controller, | |
$ | funcname, | |||
$ | page_data | |||
) | [protected] |
Invokes given action function on given controller.
- Parameters:
-
IController $controller The controller to invoke action upon string $funcname The function to invoke PageData $page_data
- Exceptions:
-
Exception if function does not exist on controller
- Returns:
- mixed Status
Reimplemented in ParameterizedRoute, and StaticPageRoute.
Definition at line 245 of file routebase.cls.php.
00245 { 00246 $this->check_action_func($controller, $funcname); 00247 return $this->controller->$funcname($page_data); 00248 }
RouteBase::is_directory | ( | ) |
Returns true, if this route is a directory (that is: ends with '/').
Implements IRoute.
Definition at line 137 of file routebase.cls.php.
00137 { 00138 return $this->is_directory; 00139 }
RouteBase::prepend_renderdecorator | ( | IRenderDecorator $ | dec | ) |
Prepend a RenderDecorator to the list of render decorators.
- Parameters:
-
IRenderDecorator $dec
- Returns:
- void
Definition at line 97 of file routebase.cls.php.
static RouteBase::split_route_id | ( | $ | route_id | ) | [static] |
Split a route identifier back into controller and action.
- Parameters:
-
string $route_id
- Returns:
- array Associative array with two keys 'controller' and 'action'
Definition at line 59 of file routebase.cls.php.
RouteBase::weight_against_path | ( | $ | path | ) |
Weight this token against path.
Implements IRoute.
Reimplemented in CatchAllRoute, ExactMatchRoute, ParameterizedRoute, and StaticPageParamterizedRoute.
Definition at line 182 of file routebase.cls.php.
00182 { 00183 //print 'WEIGHT: ' . $this->path . ' against ' . $path . ':'; 00184 00185 $ret = self::WEIGHT_NO_MATCH; 00186 if (String::starts_with($path, $this->path)) { 00187 $tmp = new PathStack($path); 00188 if ($tmp->adjust($this->path)) { 00189 $ret = $tmp->count_front(); 00190 } 00191 } 00192 00193 //print $ret .'<br />'; 00194 return $ret; 00195 }
Member Data Documentation
RouteBase::$action = ''
[protected] |
Definition at line 19 of file routebase.cls.php.
RouteBase::$controller = null
[protected] |
Definition at line 18 of file routebase.cls.php.
RouteBase::$decorators = null
[protected] |
Definition at line 22 of file routebase.cls.php.
RouteBase::$is_directory = false
[protected] |
Definition at line 23 of file routebase.cls.php.
RouteBase::$path = ''
[protected] |
Definition at line 20 of file routebase.cls.php.
RouteBase::$scheme = ''
[protected] |
Definition at line 21 of file routebase.cls.php.
The documentation for this class was generated from the following file:
- gyro/core/controller/base/routes/routebase.cls.php