ParameterizedRoute Class Reference
[Controller]
Allows defining routes that contain wildcards. More...
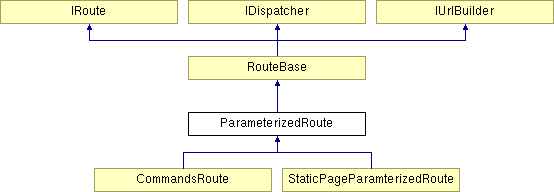
Public Member Functions |
|
weight_against_path ($path) | |
Weight this token against path. |
|
Static Public Member Functions |
|
static | add_handler (IParameterizedRouteHandler $handler) |
Add a handler. |
|
static | init_handlers () |
Autoload handlers. |
|
Protected Member Functions |
|
build_unknown_type_regexp ($type, $params) | |
Can be overloaded to support further types.
|
|
build_url_path ($params) | |
Build the URL (except base part). |
|
call_user_func_named ($controller, $function, $page_data, $params) | |
Invoke given function on controller with
given params. |
|
get_handler ($type) | |
Return handler for given type. |
|
get_handler_for_key ($key) | |
Return handler for given key. |
|
get_types () | |
Return array of types per param. |
|
initialize_adjust_path ($page_data) | |
Initialize the data passed. |
|
invoke_action_func ($controller, $funcname, $page_data) | |
Invokes given action function on given
controller. |
|
preprocess_replace_value ($value) | |
Preprocess a value before it gets inserted
into URL. |
|
replace_path_variable ($path, $key, $value) | |
Replace a variable in the path with a given
value. |
Detailed Description
Allows defining routes that contain wildcards.
A simple example: user/{id:ui>}/{page:ui}.html!
This will extract $id and $page from the URL and pass it as named variables to the according controller action:
public function action_user($page_data, $id, $page = 0);
You may also use parameters that are not handled by your actions. They will be silently ignored, when the action is called.
The wildcards are of type name[:type][:params] where type is optional and defauls to string. Params default to FALSE
The following types are supported:
i: integer ui: unsigned integer ui>: integer > 0 s: string (default). You can specify a length like so: s:2 - String of two ANSI characters sp: ASCII string. Value gets converted to plain ascii before substituting it. You can specify a length like so: sp:2. e: enum. Usage is e:value1,value2,value3,..,valueN
Two meta types are available:
_class_: Gets replaced by the class name of an instance passed as parameter _ model_: Gets replaced by te model name of an instance passed as parameter
Both meta types cannot be bound to variables. You must use the string type "s"
/user/{id:ui>}/{name:sp&}/
The action function only must handle the id parameter. You should validate the URL using
ActionHandler::validate_against_current()
to avoid different URL for the same content.
The parametrized route supports optional elements, but only at the end of the query
A terminating ! means this part of the URL is optional. Be sure to define a default value in the controller action called.
You may use the % to indicate arbitrary further path elements, or * if you want arbitrary many elements that additionally are optional.
This definition would match any URL that starts with "user" and an ID:
user/{id:ui>}/{path:s}*
For example
- user/5/profile/images would be translated to id = 5 and path = 'profile/images'
- user/5/ would be translated to id = 5 and path = ''
This definition would behave different:
user/{id:ui>}/{path:s}%
For example
- user/5/profile/images would be translated to id = 5 and path = 'profile/images'
- user/5/ would be causing a 404 not found, unless you defined another matching route
If you use the exclamation mark, only one element is allowed
user/{id:ui>}/{path:s}!
- user/5/profile would be translated to id = 5 and path = 'profile'
- user/5/profile/images would be causing a 404 not found, unless you defined another matching route
- user/5/ would be translated to id = 5 and path = ''
Definition at line 93 of file parameterizedroute.cls.php.
Member Function Documentation
static ParameterizedRoute::add_handler | ( | IParameterizedRouteHandler $ | handler | ) | [static] |
Add a handler.
Definition at line 479 of file parameterizedroute.cls.php.
ParameterizedRoute::build_unknown_type_regexp | ( | $ | type, | |
$ | params | |||
) | [protected] |
Can be overloaded to support further types.
- Parameters:
-
string $type The type of an expression string $params Optional params
- Returns:
- string A regular expression
Definition at line 334 of file parameterizedroute.cls.php.
00334 { 00335 throw new Exception(tr('Illegal expression type found: %t', 'core', array('%t' => $type))); 00336 }
ParameterizedRoute::build_url_path | ( | $ | params | ) | [protected] |
Build the URL (except base part).
- Parameters:
-
mixed $params Further parameters to use to build URL
- Returns:
- string
Reimplemented from RouteBase.
Definition at line 390 of file parameterizedroute.cls.php.
00390 { 00391 $path = $this->path; 00392 if (is_array($params) || is_object($params)) { 00393 foreach($params as $key => $value) { 00394 $path = $this->replace_path_variable($path, $key, $value); 00395 } 00396 } 00397 if (is_object($params)) { 00398 $path = $this->replace_path_variable($path, '_class_', get_class($params)); 00399 if ($params instanceof IDBTable) { 00400 $path = $this->replace_path_variable($path, '_model_', $params->get_table_name()); 00401 } 00402 } 00403 00404 // replace optional 00405 $reg_optional_asterix = '#\{[^\}]*\}\*#'; 00406 $reg_optional_exklamation = '#\{[^\}]*\}\!#'; 00407 $path = preg_replace($reg_optional_asterix, '', $path); 00408 $path = preg_replace($reg_optional_exklamation, '', $path); 00409 00410 return $path; 00411 }
ParameterizedRoute::call_user_func_named | ( | $ | controller, | |
$ | function, | |||
$ | page_data, | |||
$ | params | |||
) | [protected] |
Invoke given function on controller with given params.
- Parameters:
-
IController $controller string $function PageData $page_data array $params
- Returns:
- mixed
Definition at line 361 of file parameterizedroute.cls.php.
00361 { 00362 $reflect = new ReflectionMethod($controller, $function); 00363 $params['page_data'] = $page_data; 00364 $real_params = array(); 00365 foreach ($reflect->getParameters() as $i => $param) { 00366 $pname = $param->getName(); 00367 if (array_key_exists($pname, $params)) { 00368 $real_params[] = $params[$pname]; 00369 } 00370 else if ($param->isDefaultValueAvailable()) { 00371 $real_params[] = $param->getDefaultValue(); 00372 } 00373 else { 00374 throw new Exception('Call to function ' . $function . ' missing parameter ' . $pname); 00375 } 00376 } 00377 return call_user_func_array(array($controller, $function), $real_params); 00378 }
ParameterizedRoute::get_handler | ( | $ | type | ) | [protected] |
Return handler for given type.
- Returns:
- IParameterizedRouteHandler
Definition at line 198 of file parameterizedroute.cls.php.
00198 { 00199 return Arr::get_item(self::$handlers, $type, false); 00200 }
ParameterizedRoute::get_handler_for_key | ( | $ | key | ) | [protected] |
Return handler for given key.
- Returns:
- IParameterizedRouteHandler
Definition at line 207 of file parameterizedroute.cls.php.
00207 { 00208 $types = $this->get_types(); 00209 $type = Arr::get_item($types, $key, 's'); 00210 return $this->get_handler($type); 00211 }
ParameterizedRoute::get_types | ( | ) | [protected] |
Return array of types per param.
- Returns:
- array
Definition at line 189 of file parameterizedroute.cls.php.
static ParameterizedRoute::init_handlers | ( | ) | [static] |
Autoload handlers.
Definition at line 464 of file parameterizedroute.cls.php.
00464 { 00465 $files = Load::get_files_from_directory('controller/base/routes/parameterizedroutehandlers', '*.handler.php'); 00466 foreach($files as $file => $inc) { 00467 include_once $inc; 00468 $cls = Load::filename_to_classname($file, 'ParameterizedRouteHandler', 'handler'); 00469 if (!class_exists($cls)) { 00470 throw new Exception('ParameterizedRoute: ' . $inc . ' does not define class ' . $cls); 00471 } 00472 self::add_handler(new $cls()); 00473 } 00474 }
ParameterizedRoute::initialize_adjust_path | ( | $ | page_data | ) | [protected] |
Initialize the data passed.
- Parameters:
-
PageData $page_data
Reimplemented from RouteBase.
Definition at line 179 of file parameterizedroute.cls.php.
ParameterizedRoute::invoke_action_func | ( | $ | controller, | |
$ | funcname, | |||
$ | page_data | |||
) | [protected] |
Invokes given action function on given controller.
- Parameters:
-
IController $controller The controller to invoke action upon string $funcname The function to invoke PageData $page_data
- Exceptions:
-
Exception if function does not exist on controller
- Returns:
- mixed Status
Reimplemented from RouteBase.
Definition at line 347 of file parameterizedroute.cls.php.
00347 { 00348 $this->check_action_func($controller, $funcname); 00349 return $this->call_user_func_named($controller, $funcname, $page_data, $this->params); 00350 }
ParameterizedRoute::preprocess_replace_value | ( | $ | value | ) | [protected] |
Preprocess a value before it gets inserted into URL.
Definition at line 453 of file parameterizedroute.cls.php.
00453 { 00454 return str_replace(array('%2F', '%3F', '%26'), array('%252F', '%253F', '%2526'), urlencode(Cast::string($value))); 00455 }
ParameterizedRoute::replace_path_variable | ( | $ | path, | |
$ | key, | |||
$ | value | |||
) | [protected] |
Replace a variable in the path with a given value.
- Parameters:
-
string $path string $key mixed $value
- Returns:
- string
Definition at line 421 of file parameterizedroute.cls.php.
00421 { 00422 if (is_object($value) || is_array($value)) { 00423 return $path; 00424 } 00425 00426 // Replace otional type with string type. Reduces RegExp complexity, 00427 // since we now can force a ":" after key and before type 00428 $path = str_replace('{' . $key . '}', '{' . $key . ':s}', $path); 00429 00430 // Figure out type of $key 00431 $reg = '#\{' . $key . ':([^:\}]*)[:]?.*?\}#'; 00432 $matches = array(); 00433 if (preg_match($reg, $path, $matches)) { 00434 $handler = $this->get_handler($matches[1]); 00435 if ($handler) { 00436 $value = $handler->preprocess_build_url($value); 00437 } 00438 } 00439 00440 $reg = '#\{' . $key . ':[^}]*\}[*%]#'; 00441 $replace = implode('/', array_map(array($this, 'preprocess_replace_value'), explode('/', Cast::string($value)))); 00442 $path = preg_replace($reg, $replace, $path); 00443 $reg = '#\{' . $key . ':[^}]*\}!?#'; 00444 $replace = $this->preprocess_replace_value($value); 00445 $path = preg_replace($reg, $replace, $path); 00446 00447 return $path; 00448 }
ParameterizedRoute::weight_against_path | ( | $ | path | ) |
Weight this token against path.
Reimplemented from RouteBase.
Reimplemented in StaticPageParamterizedRoute.
Definition at line 122 of file parameterizedroute.cls.php.
00122 { 00123 $def_pathstack = new PathStack($this->path); // Definition 00124 $url_pathstack = new PathStack($path); // URL to process 00125 00126 $ret = self::WEIGHT_NO_MATCH; 00127 // Do some simple plausibility check 00128 $simple_check = true; 00129 $cf_delta = $url_pathstack->count_front() - $def_pathstack->count_front(); 00130 $lastchar = substr(rtrim($this->path, '/'), -1); 00131 switch ($lastchar) { 00132 case '!': 00133 $simple_check = ($cf_delta == 0 || $cf_delta == -1); 00134 break; 00135 case '%': 00136 $simple_check = ($cf_delta >= 0); 00137 break; 00138 case '*': 00139 $simple_check = ($cf_delta >= -1); 00140 break; 00141 default: 00142 $simple_check = ($cf_delta == 0); 00143 break; 00144 } 00145 00146 if ($simple_check) { 00147 $ret = self::WEIGHT_FULL_MATCH; 00148 $count = 0; 00149 while ($def_elem = $def_pathstack->shift()) { 00150 $count++; 00151 $url_elem = ''; 00152 switch (substr($def_elem, -1)) { 00153 case '*': 00154 case '%': 00155 //$ret = $count; 00156 $url_elem = $url_pathstack->implode_front(); 00157 $url_pathstack->clear_front(); 00158 break; 00159 default: 00160 $url_elem = $url_pathstack->shift(); 00161 break; 00162 } 00163 00164 if ($this->validate($def_elem, $url_elem) == false) { 00165 $ret = self::WEIGHT_NO_MATCH; 00166 break; 00167 } 00168 } 00169 } 00170 00171 return $ret; 00172 }
The documentation for this class was generated from the following file:
- gyro/core/controller/base/routes/parameterizedroute.cls.php