DBQuery Class Reference
[Model]
Represents a DB query. More...
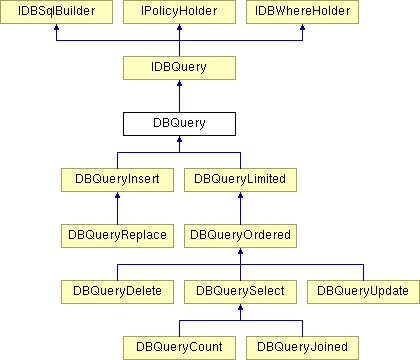
Public Member Functions |
|
__construct (IDBTable $table, $policy=self::NORMAL) | |
add_field ($field) | |
Add a field. |
|
add_where ($column, $operator=null, $value=null, $mode=IDBWhere::LOGIC_AND) | |
Adds where to this. |
|
add_where_object (IDBWhere $where) | |
Adds IDBWhere instance
to this. |
|
get_fields () | |
Return fields. |
|
get_policy () | |
Returns policy. |
|
get_table () | |
Returns table. |
|
get_wheres () | |
Returns root collection of wheres. |
|
has_policy ($policy) | |
Returns true, if has given policy. |
|
set_fields ($arr_fields) | |
Set the fields this query affects. |
|
set_policy ($policy) | |
Set policy. |
|
Protected Attributes |
|
$fields = array() | |
$policy | |
$table | |
$wheres |
Detailed Description
Represents a DB query.
Definition at line 8 of file dbquery.cls.php.
Constructor & Destructor Documentation
DBQuery::__construct | ( | IDBTable $ | table, | |
$ | policy =
self::NORMAL |
|||
) |
Definition at line 34 of file dbquery.cls.php.
00034 { 00035 $this->table = $table; 00036 $this->policy = $policy; 00037 $this->wheres = new DBWhereGroup($table); 00038 }
Member Function Documentation
DBQuery::add_field | ( | $ | field | ) |
Add a field.
- Parameters:
-
mixed $field
Implements IDBQuery.
Definition at line 91 of file dbquery.cls.php.
DBQuery::add_where | ( | $ | column, | |
$ | operator = null , |
|||
$ | value = null , |
|||
$ | mode = IDBWhere::LOGIC_AND |
|||
) |
Adds where to this.
- Parameters:
-
string $column Column to query upon, or a full sql where statement string $operator Operator to execute mixed $value Value(s) to use string $mode Either IDBWhere::LOGIC_AND or IDBWhere::LOGIC_OR
Implements IDBWhereHolder.
Definition at line 140 of file dbquery.cls.php.
DBQuery::add_where_object | ( | IDBWhere $ | where | ) |
Adds IDBWhere instance to this.
Implements IDBWhereHolder.
Definition at line 147 of file dbquery.cls.php.
DBQuery::get_fields | ( | ) |
DBQuery::get_policy | ( | ) |
Returns policy.
- Returns:
- int
Implements IPolicyHolder.
Definition at line 118 of file dbquery.cls.php.
DBQuery::get_table | ( | ) |
Returns table.
- Returns:
- IDBTable
Implements IDBQuery.
Definition at line 45 of file dbquery.cls.php.
DBQuery::get_wheres | ( | ) |
Returns root collection of wheres.
- Returns:
- DBWhereGroup
Implements IDBWhereHolder.
Reimplemented in DBQuerySelect.
Definition at line 54 of file dbquery.cls.php.
DBQuery::has_policy | ( | $ | policy | ) |
Returns true, if has given policy.
- Parameters:
-
int $policy
- Returns:
- bool
Implements IPolicyHolder.
Definition at line 128 of file dbquery.cls.php.
00128 { 00129 return Common::flag_is_set($this->get_policy(), $policy); 00130 }
DBQuery::set_fields | ( | $ | arr_fields | ) |
Set the fields this query affects.
Dependent on the query time, the array passed can be of different forms
UPDATE and INSERT: Associative array with field name as key and field value as value
$query = new DBQuery($some_table); $query->set_fields(array('name' => 'Johnny')); $query->add_where('id', '=', 3); $sql = $query->build_update_sql(); // returns UPDATE some_table SET name = 'Johnny' WHERE id = 3
SELECT: Either array of field names or associative array with field name as key and field alias as value. Both can be combined.
$query = new DBQuery($some_table); $query->set_fields(array('count(name)' => 'c', 'phone')); $sql = $query->build_select_sql(); // returns SELECT count(name) AS c, phone FROM some_table
If invoked with DBQuery::CLEAR, the fields will get cleared
- Parameters:
-
array $arr_fields
- Returns:
- void
Implements IDBQuery.
Definition at line 82 of file dbquery.cls.php.
00082 { 00083 $this->fields = Arr::force($arr_fields, false); 00084 }
DBQuery::set_policy | ( | $ | policy | ) |
Set policy.
- Parameters:
-
int $policy Either DBQuerySelect::DEFAULT_POLICY or DBQuerySelect::DISTINCT_POLICY
Implements IPolicyHolder.
Definition at line 109 of file dbquery.cls.php.
00109 { 00110 $this->policy = $policy; 00111 }
Member Data Documentation
DBQuery::$fields = array()
[protected] |
Definition at line 26 of file dbquery.cls.php.
DBQuery::$policy [protected] |
Definition at line 32 of file dbquery.cls.php.
DBQuery::$table [protected] |
Definition at line 14 of file dbquery.cls.php.
DBQuery::$wheres [protected] |
Definition at line 20 of file dbquery.cls.php.
The documentation for this class was generated from the following file:
- gyro/core/model/base/dbquery.cls.php