WidgetTree Class Reference
A widgets that prints a tree, consisting of nested More...
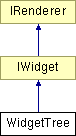
Public Member Functions |
|
__construct ($params) | |
render ($policy=self::NONE) | |
Static Public Member Functions |
|
static | output ($params, $policy=self::OPEN_LEAF) |
Public Attributes |
|
$params = array() | |
const | OPEN_LEAF = 1024 |
const | OPEN_UNTIL_LEVEL = 512 |
const | P_ACTION = 'action' |
Action to use to create links. |
|
const | P_LEAF = 'leaf' |
"leaf" parameter name |
|
const | P_LEVEL = 'level' |
"level" parameter name |
|
const | P_ROOTS = 'roots' |
"roots" parameter name |
|
Protected Member Functions |
|
build_branch_array ($params, $policy) | |
Build branch of leaf, that is chain of leaf
and leaf's parent. |
|
build_tree_array ($params, $policy, $branch) | |
Build the tree. |
|
create_level ($level, $max_level, $items, $policy, $branch) | |
Creates a level that is a set of child
nodes. |
|
create_node ($item, $childs, $is_branch, $is_leaf) |
Detailed Description
A widgets that prints a tree, consisting of nested
Definition at line 5 of file tree.widget.php.
Constructor & Destructor Documentation
WidgetTree::__construct | ( | $ | params | ) |
Definition at line 33 of file tree.widget.php.
00033 { 00034 $this->params = $params; 00035 }
Member Function Documentation
WidgetTree::build_branch_array | ( | $ | params, | |
$ | policy | |||
) | [protected] |
Build branch of leaf, that is chain of leaf and leaf's parent.
Definition at line 52 of file tree.widget.php.
00052 { 00053 $ret = array(); 00054 $leaf = Arr::get_item($params, self::P_LEAF, false); 00055 if ($leaf) { 00056 $item = $leaf; 00057 while($item) { 00058 array_unshift($ret, $item); 00059 $item = $item->get_parent(); 00060 } 00061 } 00062 return $ret; 00063 }
WidgetTree::build_tree_array | ( | $ | params, | |
$ | policy, | |||
$ | branch | |||
) | [protected] |
Build the tree.
Definition at line 68 of file tree.widget.php.
00068 { 00069 $max_level = Common::flag_is_set($policy, self::OPEN_UNTIL_LEVEL) ? Arr::get_item($params, self::P_LEVEL, 0) : 0; 00070 $roots = Arr::force(Arr::get_item($params, self::P_ROOTS, array()), false); 00071 return $this->create_level(0, $max_level, $roots, $policy, $branch); 00072 }
WidgetTree::create_level | ( | $ | level, | |
$ | max_level, | |||
$ | items, | |||
$ | policy, | |||
$ | branch | |||
) | [protected] |
Creates a level that is a set of child nodes.
- Parameters:
-
int $level Deepness of level int $max_level Maximum levels to be expanded by default array $items Nodes of parent level int $policy Render policy array $branch Elements that for a branch to a given leaf. Branches always get expanded
Definition at line 83 of file tree.widget.php.
00083 { 00084 $ret = array(); 00085 // If has next level, create a next level 00086 $has_next_level = ($level < $max_level); 00087 // Extract current branch item 00088 $branch_item = array_shift($branch); 00089 foreach($items as $item) { 00090 // $is_branch indicates if a branch is processed 00091 $is_branch = ($branch_item) ? $item->is_same_as($branch_item) : false; 00092 // True if last element on branch is reached 00093 $is_leaf = $is_branch && (count($branch) == 0); 00094 if ($is_branch) { 00095 // A branch is always expanded up to leaf 00096 // But expand leaf itself only if render policy states so 00097 $expand_branch = (!$is_leaf || Common::flag_is_set($policy, self::OPEN_LEAF)); 00098 if ($expand_branch) { 00099 $childs = $this->create_level($level + 1, $max_level, $item->get_childs(), $policy, $branch); 00100 } 00101 } 00102 else if ($has_next_level) { 00103 // Expand next level that is not a branch 00104 $childs = $this->create_level($level + 1, $max_level, $item->get_childs(), $policy, array()); 00105 } 00106 else { 00107 $childs = array(); 00108 } 00109 $ret[] = $this->create_node($item, $childs, $is_branch, $is_leaf); 00110 } 00111 return $ret; 00112 }
WidgetTree::create_node | ( | $ | item, | |
$ | childs, | |||
$ | is_branch, | |||
$ | is_leaf | |||
) | [protected] |
Definition at line 114 of file tree.widget.php.
static WidgetTree::output | ( | $ | params, | |
$ | policy =
self::OPEN_LEAF |
|||
) | [static] |
Definition at line 28 of file tree.widget.php.
00028 { 00029 $w = new WidgetTree($params); 00030 return $w->render($policy); 00031 }
WidgetTree::render | ( | $ | policy =
self::NONE |
) |
Implements IRenderer.
Definition at line 37 of file tree.widget.php.
00037 { 00038 // First build an array with type/id as key and title, url and childs as members 00039 $branch = $this->build_branch_array($this->params, $policy); 00040 $tree = $this->build_tree_array($this->params, $policy, $branch); 00041 00042 $view = ViewFactory::create_view(IViewFactory::MESSAGE, 'widgets/tree'); 00043 $view->assign('tree', $tree); 00044 $view->assign('action', Arr::get_item($this->params, self::P_ACTION, 'view')); 00045 $view->assign('params', $this->params); 00046 return $view->render(); 00047 }
Member Data Documentation
WidgetTree::$params = array() |
Definition at line 26 of file tree.widget.php.
const WidgetTree::OPEN_LEAF = 1024 |
Definition at line 7 of file tree.widget.php.
const WidgetTree::OPEN_UNTIL_LEVEL = 512 |
Definition at line 6 of file tree.widget.php.
const WidgetTree::P_ACTION = 'action' |
Action to use to create links.
Definition at line 24 of file tree.widget.php.
const WidgetTree::P_LEAF = 'leaf' |
"leaf" parameter name
Definition at line 16 of file tree.widget.php.
const WidgetTree::P_LEVEL = 'level' |
"level" parameter name
Definition at line 12 of file tree.widget.php.
const WidgetTree::P_ROOTS = 'roots' |
"roots" parameter name
Definition at line 20 of file tree.widget.php.
The documentation for this class was generated from the following file:
- contributions/widgets.tree/view/widgets/tree.widget.php