DBSession Class Reference
[Model]
Redirect session to write to DB. More...
Inheritance diagram for DBSession:
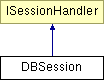
Public Member Functions |
|
close () | |
Close a session. |
|
destroy ($key) | |
Delete a session. |
|
gc ($lifetime) | |
Delete outdated sessions. |
|
open ($save_path, $session_name) | |
Open a session. |
|
read ($key) | |
Load session data from
database. |
|
write ($key, $value) | |
Write session data to DB.
|
Detailed Description
Redirect session to write to DB.
Definition at line 10 of file dbsession.cls.php.
Member Function Documentation
DBSession::close | ( | ) |
Close a session.
Implements ISessionHandler.
Definition at line 21 of file dbsession.cls.php.
00021 { 00022 //Note that for security reasons the Debian and Ubuntu distributions of 00023 //php do not call _gc to remove old sessions, but instead run /etc/cron.d/php*, 00024 //which check the value of session.gc_maxlifetime in php.ini and delete the session 00025 //files in /var/lib/php*. This is all fine, but it means if you write your own 00026 //session handlers you'll need to explicitly call your _gc public function yourself. 00027 //A good place to do this is in your _close public function 00028 00029 $this->gc(ini_get('session.gc_maxlifetime')); 00030 return true; 00031 }
DBSession::destroy | ( | $ | key | ) |
Delete a session.
Implements ISessionHandler.
Definition at line 77 of file dbsession.cls.php.
00077 { 00078 try { 00079 $sess = new DAOSessions(); 00080 $sess->id = $key; 00081 $sess->delete(); 00082 } 00083 catch(Exception $ex) {} 00084 }
DBSession::gc | ( | $ | lifetime | ) |
Delete outdated sessions.
Implements ISessionHandler.
Definition at line 89 of file dbsession.cls.php.
00089 { 00090 if (!Session::is_started()) { 00091 return; 00092 } 00093 try { 00094 $sess = new DAOSessions(); 00095 $sess->add_where('modificationdate', '<', time() - $lifetime); 00096 $sess->delete(DataObjectBase::WHERE_ONLY); 00097 } 00098 catch (Exception $ex) {} 00099 00100 return true; 00101 }
DBSession::open | ( | $ | save_path, | |
$ | session_name | |||
) |
DBSession::read | ( | $ | key | ) |
Load session data from database.
Implements ISessionHandler.
Definition at line 36 of file dbsession.cls.php.
00036 { 00037 // Write and Close handlers are called after destructing objects since PHP 5.0.5 00038 // Thus destructors can use sessions but session handler can't use objects. 00039 // So we are moving session closure before destructing objects. 00040 register_shutdown_function('session_write_close'); 00041 $sess = DB::get_item('sessions', 'id', $key); 00042 if ($sess) { 00043 return $sess->data; 00044 } 00045 return ''; 00046 }
DBSession::write | ( | $ | key, | |
$ | value | |||
) |
Write session data to DB.
Implements ISessionHandler.
Definition at line 51 of file dbsession.cls.php.
00051 { 00052 try { 00053 // Rollback any open transactions, if there are any 00054 //DB::rollback(); 00055 $sess = DB::get_item('sessions', 'id', $key); 00056 $err = false; 00057 if ($sess) { 00058 $sess->data = $value; 00059 $err = $sess->update(); 00060 } 00061 else { 00062 $sess = new DAOSessions(); 00063 $sess->id = $key; 00064 $sess->data = $value; 00065 $err = $sess->insert(); 00066 } 00067 return $err->is_ok(); 00068 } 00069 catch(Exception $ex) { 00070 return false; 00071 } 00072 }
The documentation for this class was generated from the following file:
- gyro/core/model/base/dbsession.cls.php