CommandComposite Class Reference
[Behaviour]
The command composite executes a set of commands. More...
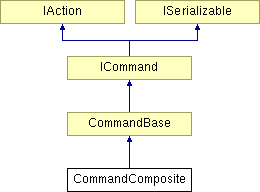
Public Member Functions |
|
append ($command) | |
Append a command to the chain. |
|
append_array ($commands) | |
Append a command array to the chain.
|
|
can_execute ($user) | |
Returns TRUE, if all commands in the chain
can be executed by given user. |
|
execute () | |
Execute all commands (until one fails.
|
|
undo () | |
Undo all commands in chain. |
|
Protected Member Functions |
|
do_can_execute ($user) | |
Does execution checking. |
|
do_execute () | |
Does executing. |
|
do_undo () | |
Does undo. |
|
undo_on_chain ($commands) | |
Undo comnmands passed. |
|
Protected Attributes |
|
$commands = array() |
Detailed Description
The command composite executes a set of commands.
Comamnds you append are executed in a tree-like manor.
This command wraps a database transaction around the execution of all commands
Definition at line 14 of file commandcomposite.cls.php.
Member Function Documentation
CommandComposite::append | ( | $ | command | ) |
Append a command to the chain.
Definition at line 20 of file commandcomposite.cls.php.
00020 { 00021 if ($command instanceof ICommand) { 00022 $this->commands[] = $command; 00023 } 00024 }
CommandComposite::append_array | ( | $ | commands | ) |
Append a command array to the chain.
Definition at line 29 of file commandcomposite.cls.php.
CommandComposite::can_execute | ( | $ | user | ) |
Returns TRUE, if all commands in the chain can be executed by given user.
Reimplemented from CommandBase.
Definition at line 40 of file commandcomposite.cls.php.
00040 { 00041 $ret = $this->do_can_execute($user); 00042 if ($ret) { 00043 foreach($this->commands as $command) { 00044 if (!$command->can_execute($user)) { 00045 return false; 00046 } 00047 } 00048 } 00049 return $ret; 00050 }
CommandComposite::do_can_execute | ( | $ | user | ) | [protected] |
Does execution checking.
- Returns:
- bool
Definition at line 108 of file commandcomposite.cls.php.
00108 { 00109 return parent::can_execute($user); 00110 }
CommandComposite::do_execute | ( | ) | [protected] |
Does executing.
- Returns:
- Status
Definition at line 117 of file commandcomposite.cls.php.
00117 { 00118 return parent::execute(); 00119 }
CommandComposite::do_undo | ( | ) | [protected] |
Does undo.
Definition at line 124 of file commandcomposite.cls.php.
00124 { 00125 parent::undo(); 00126 }
CommandComposite::execute | ( | ) |
Execute all commands (until one fails.
.)
Reimplemented from CommandBase.
Definition at line 55 of file commandcomposite.cls.php.
00055 { 00056 $ret = new Status(); 00057 $arr_executed = array(); 00058 DB::start_trans(); 00059 try { 00060 $ret = $this->do_execute(); 00061 if ($ret->is_ok()) { 00062 foreach($this->commands as $command) { 00063 $ret->merge($command->execute()); 00064 if ($ret->is_error()) { 00065 // Ups, something went wrong 00066 break; 00067 } 00068 $arr_executed[] = $command; 00069 } 00070 } 00071 } 00072 catch (Exception $ex) { 00073 $ret->merge($ex); 00074 } 00075 00076 if ($ret->is_error()) { 00077 $this->undo_on_chain($arr_executed); 00078 $this->undo(); 00079 } 00080 DB::end_trans($ret); 00081 return $ret; 00082 }
CommandComposite::undo | ( | ) |
Undo all commands in chain.
Reimplemented from CommandBase.
Definition at line 87 of file commandcomposite.cls.php.
00087 { 00088 $this->do_undo(); 00089 $this->undo_on_chain($this->commands); 00090 }
CommandComposite::undo_on_chain | ( | $ | commands | ) | [protected] |
Undo comnmands passed.
Definition at line 95 of file commandcomposite.cls.php.
Member Data Documentation
CommandComposite::$commands = array()
[protected] |
Definition at line 15 of file commandcomposite.cls.php.
The documentation for this class was generated from the following file:
- gyro/core/behaviour/base/commandcomposite.cls.php