CommandChain Class Reference
[Behaviour]
The command chain executes commands that form a hierarchy. More...
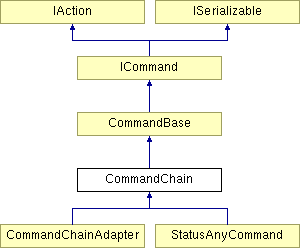
Public Member Functions |
|
append ($cmd) | |
Set the next command in chain. |
|
append_array ($commands) | |
Append a command array to the chain.
|
|
can_execute ($user) | |
Returns TRUE, if the command can be executed
by given user. |
|
execute () | |
Executes commands. |
|
undo () | |
Revert execution. |
|
Protected Member Functions |
|
do_can_execute ($user) | |
Does execution checking. |
|
do_execute () | |
Does executing. |
|
do_undo () | |
Does undo. |
|
get_next () | |
Return next command. |
|
get_prev () | |
Return previous command. |
|
set_prev ($cmd) | |
Set previous command. |
|
Protected Attributes |
|
$next = null | |
$prev = null |
Detailed Description
The command chain executes commands that form a hierarchy.
Every command you append is put at the end of the chain
This command wraps a database transaction around the execution of all commands
Definition at line 14 of file commandchain.cls.php.
Member Function Documentation
CommandChain::append | ( | $ | cmd | ) |
Set the next command in chain.
Definition at line 104 of file commandchain.cls.php.
00104 { 00105 if ($this->next) { 00106 $this->next->append($cmd); 00107 } 00108 else { 00109 if ($cmd instanceof ICommand) { 00110 if ($cmd instanceof CommandChain) { 00111 $this->next = $cmd; 00112 $cmd->set_prev($this); 00113 } 00114 else { 00115 $adapter = new CommandChainAdapter($cmd); 00116 $this->append($adapter); 00117 } 00118 } 00119 } 00120 }
CommandChain::append_array | ( | $ | commands | ) |
Append a command array to the chain.
Definition at line 125 of file commandchain.cls.php.
00125 { 00126 if (is_array($commands)) { 00127 foreach($commands as $command) { 00128 $this->append($command); 00129 } 00130 } 00131 }
CommandChain::can_execute | ( | $ | user | ) |
Returns TRUE, if the command can be executed by given user.
Reimplemented from CommandBase.
Definition at line 31 of file commandchain.cls.php.
00031 { 00032 $ret = $this->do_can_execute($user); 00033 if ($ret) { 00034 $next = $this->get_next(); 00035 if ($next) { 00036 $ret = $next->can_execute($user); 00037 } 00038 } 00039 return $ret; 00040 }
CommandChain::do_can_execute | ( | $ | user | ) | [protected] |
Does execution checking.
Reimplemented in CommandChainAdapter, and StatusAnyCommand.
Definition at line 145 of file commandchain.cls.php.
00145 { 00146 return parent::can_execute($user); 00147 }
CommandChain::do_execute | ( | ) | [protected] |
Does executing.
Reimplemented in CommandChainAdapter, and StatusAnyCommand.
Definition at line 152 of file commandchain.cls.php.
00152 { 00153 return parent::execute(); 00154 }
CommandChain::do_undo | ( | ) | [protected] |
Does undo.
Reimplemented in CommandChainAdapter.
Definition at line 159 of file commandchain.cls.php.
00159 { 00160 parent::undo(); 00161 }
CommandChain::execute | ( | ) |
Executes commands.
- Returns:
- Status
Reimplemented from CommandBase.
Definition at line 47 of file commandchain.cls.php.
00047 { 00048 $ret = new Status(); 00049 00050 DB::start_trans(); 00051 try { 00052 $ret = $this->do_execute(); 00053 if ($ret->is_ok()) { 00054 $next = $this->get_next(); 00055 if ($next) { 00056 $ret = $next->execute(); 00057 } 00058 } 00059 } 00060 catch (Exception $ex) { 00061 $ret->merge($ex); 00062 } 00063 00064 if ($ret->is_ok()) { 00065 $ret->merge(parent::execute()); 00066 } 00067 00068 if ($ret->is_error()) { 00069 $this->undo(); 00070 } 00071 DB::end_trans($ret); 00072 00073 return $ret; 00074 }
CommandChain::get_next | ( | ) | [protected] |
Return next command.
Definition at line 90 of file commandchain.cls.php.
CommandChain::get_prev | ( | ) | [protected] |
Return previous command.
Definition at line 97 of file commandchain.cls.php.
CommandChain::set_prev | ( | $ | cmd | ) | [protected] |
Set previous command.
Definition at line 136 of file commandchain.cls.php.
00136 { 00137 if ($cmd instanceof ICommand) { 00138 $this->prev = $cmd; 00139 } 00140 }
CommandChain::undo | ( | ) |
Revert execution.
Reimplemented from CommandBase.
Definition at line 79 of file commandchain.cls.php.
Member Data Documentation
CommandChain::$next = null
[protected] |
Definition at line 20 of file commandchain.cls.php.
CommandChain::$prev = null
[protected] |
Definition at line 26 of file commandchain.cls.php.
The documentation for this class was generated from the following file:
- gyro/core/behaviour/base/commandchain.cls.php