AccessControlBase Class Reference
[Behaviour]
Base class for Access Control Cheching. More...
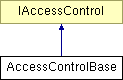
Public Member Functions |
|
__construct ($item_types=false) | |
Pass types (=model names), the
implementation is responsible of. |
|
is_allowed ($action, $item, $user, $params=false) | |
Check if action on object is allowed for
user. |
|
set_old_implementation (IAccessControl $implementation) | |
Set old implementation. |
|
Public Attributes |
|
const | ALLOWED = 1 |
If returned by do_is_allowed() functions, will set
return value of is_allowed()
to true. |
|
const | NOT_ALLOWED = 0 |
If returned by do_is_allowed() functions, will set
return value of is_allowed()
to false. |
|
const | NOT_RESPONSIBLE = -1 |
If returned by do_is_allowed() functions, will force
delegate to be called (if any). |
|
Protected Member Functions |
|
do_is_allowed ($action, $item, $user, $params=false) | |
Overloadable. |
|
do_is_allowed_for_anonymous ($action, $item, $params=false) | |
Overloadable. |
|
do_is_allowed_for_user ($action, $item, $user, $params=false) | |
Overloadable. |
|
get_item_type ($item) | |
Returns type of item. |
|
to_result ($bool) | |
Convert Bool to tri-state. |
Detailed Description
Base class for Access Control Cheching.
Definition at line 7 of file accesscontrolbase.cls.php.
Constructor & Destructor Documentation
AccessControlBase::__construct | ( | $ | item_types = false |
) |
Pass types (=model names), the implementation is responsible of.
- Parameters:
-
string|array $item_types
Definition at line 39 of file accesscontrolbase.cls.php.
00039 { 00040 if (!empty($item_types)) { 00041 $this->types = Arr::force($item_types, false); 00042 } 00043 }
Member Function Documentation
AccessControlBase::do_is_allowed | ( | $ | action, | |
$ | item, | |||
$ | user, | |||
$ | params = false |
|||
) | [protected] |
Overloadable.
Check if action on object is allowed for user
- Parameters:
-
string $action The action to perform (edit, delete, ....) mixed $item Item to perform the action on (may be a DataObject, e.g.) mixed $user A user, role, ACO, depending on user management chosen
- Returns:
- int One of Constants ALLOWED, NOT_ALLOWED and NOT_RESPONSIBLE
Definition at line 80 of file accesscontrolbase.cls.php.
00080 { 00081 // Check type based responsibility 00082 if (!empty($this->types)) { 00083 $resposible = false; 00084 $item_type = $this->get_item_type($item); 00085 foreach($this->types as $type) { 00086 if ($type === $item_type) { 00087 $resposible = true; 00088 break; 00089 } 00090 } 00091 if (!$resposible) { 00092 return self::NOT_RESPONSIBLE; 00093 } 00094 } 00095 00096 // We are responsible 00097 if (!empty($user)) { 00098 return $this->do_is_allowed_for_user($action, $item, $user, $params); 00099 } 00100 else { 00101 return $this->do_is_allowed_for_anonymous($action, $item, $params); 00102 } 00103 }
AccessControlBase::do_is_allowed_for_anonymous | ( | $ | action, | |
$ | item, | |||
$ | params = false |
|||
) | [protected] |
Overloadable.
Check if action on object is allowed for no user
User is always valid
- Parameters:
-
string $action The action to perform (edit, delete, ....) mixed $item Item to perform the action on (may be a DataObject, e.g.)
- Returns:
- int One of Constants ALLOWED, NOT_ALLOWED and NOT_RESPONSIBLE
Definition at line 128 of file accesscontrolbase.cls.php.
AccessControlBase::do_is_allowed_for_user | ( | $ | action, | |
$ | item, | |||
$ | user, | |||
$ | params = false |
|||
) | [protected] |
Overloadable.
Check if action on object is allowed for given user
User is always valid
- Parameters:
-
string $action The action to perform (edit, delete, ....) mixed $item Item to perform the action on (may be a DataObject, e.g.) mixed $user A user, role, ACO, depending on user management chosen
- Returns:
- int One of Constants ALLOWED, NOT_ALLOWED and NOT_RESPONSIBLE
Definition at line 115 of file accesscontrolbase.cls.php.
AccessControlBase::get_item_type | ( | $ | item | ) | [protected] |
Returns type of item.
- Parameters:
-
mixed $item
- Returns:
- string
Definition at line 148 of file accesscontrolbase.cls.php.
00148 { 00149 $ret = $item; 00150 if ($item instanceof IDBTable) { 00151 $ret = $item->get_table_name(); 00152 } 00153 else if (is_object($item)) { 00154 $ret = get_class($item); 00155 } 00156 else if (is_null($item)) { 00157 $ret = ''; 00158 } 00159 return $ret; 00160 }
AccessControlBase::is_allowed | ( | $ | action, | |
$ | item, | |||
$ | user, | |||
$ | params = false |
|||
) |
Check if action on object is allowed for user.
- Parameters:
-
string $action The action to perform (edit, delete, ....) mixed $item Item to perform the action on (may be a DataObject, e.g.) mixed $user A user, role, ACO, depending on user management chosen
- Returns:
- bool
Implements IAccessControl.
Definition at line 53 of file accesscontrolbase.cls.php.
00053 { 00054 $ret = false; 00055 $result = $this->do_is_allowed($action, $item, $user, $params); 00056 if ($result === true) { 00057 $result = self::ALLOWED; 00058 } 00059 switch ($result) { 00060 case self::NOT_RESPONSIBLE: 00061 if ($this->delegate) { 00062 $ret = $this->delegate->is_allowed($action, $item, $user, $params); 00063 } 00064 break; 00065 default: 00066 $ret = ($result) ? true : false; 00067 break; 00068 } 00069 return $ret; 00070 }
AccessControlBase::set_old_implementation | ( | IAccessControl $ | implementation | ) |
Set old implementation.
Requests not handled should be delegated to this
- Parameters:
-
IAccessControl $implementation
Implements IAccessControl.
Definition at line 167 of file accesscontrolbase.cls.php.
AccessControlBase::to_result | ( | $ | bool | ) | [protected] |
Convert Bool to tri-state.
- Parameters:
-
bool $bool
- Returns:
- int
Definition at line 138 of file accesscontrolbase.cls.php.
Member Data Documentation
const AccessControlBase::ALLOWED = 1 |
If returned by do_is_allowed() functions, will set return value of is_allowed() to true.
Definition at line 28 of file accesscontrolbase.cls.php.
const AccessControlBase::NOT_ALLOWED = 0 |
If returned by do_is_allowed() functions, will set return value of is_allowed() to false.
Definition at line 24 of file accesscontrolbase.cls.php.
const AccessControlBase::NOT_RESPONSIBLE = -1 |
If returned by do_is_allowed() functions, will force delegate to be called (if any).
Definition at line 32 of file accesscontrolbase.cls.php.
The documentation for this class was generated from the following file:
- gyro/core/behaviour/base/accesscontrolbase.cls.php